Vue3和Typescript怎么配合使用
时间: 2023-11-28 22:47:24 浏览: 95
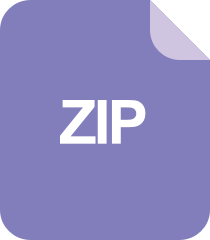
vue3-typescript
Vue3 和 TypeScript 配合使用可以提高代码的可读性、可维护性和健壮性。下面我给你提供一些配合使用的方法:
1. 使用 Vue CLI 创建项目时,选择 TypeScript 选项,这样就可以自动生成 TypeScript 的配置文件,并且在编写组件时也能享受到 TypeScript 的类型检查。
2. 在组件中使用 TypeScript,可以先定义组件的 props 和 data 的类型,这样在编写组件时就能享受到 TypeScript 的类型检查,例如:
```typescript
<template>
<div>{{ message }}</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
export default defineComponent({
props: {
name: {
type: String,
required: true
}
},
data() {
return {
message: `Hello ${this.name}!`
}
}
})
</script>
```
3. 在 Vue3 中,使用 Composition API 编写组件时,也可以结合 TypeScript 使用,例如:
```typescript
<template>
<div>{{ message }}</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue'
export default defineComponent({
props: {
name: {
type: String,
required: true
}
},
setup(props) {
const message = ref(`Hello ${props.name}!`)
return {
message
}
}
})
</script>
```
4. 如果你使用了第三方库,并且该库没有提供类型声明文件,可以手动创建一个类型声明文件,例如:
```typescript
// my-library.d.ts
declare module 'my-library' {
export function myFunction(): void
}
```
这样在使用该库时就能享受到 TypeScript 的类型检查。
总之,Vue3 和 TypeScript 配合使用可以提高代码的可读性、可维护性和健壮性,建议在项目中使用。
阅读全文
相关推荐
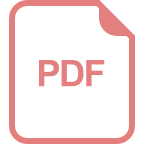
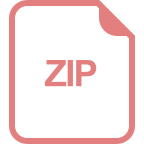
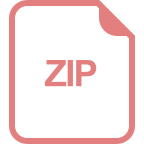
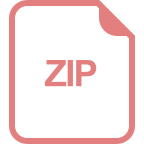
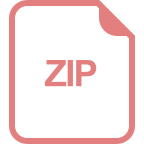
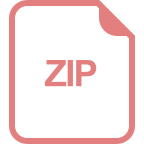
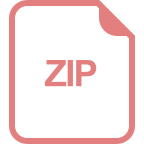
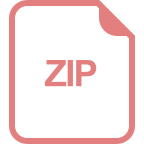
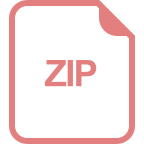
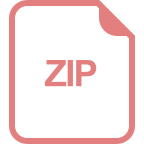
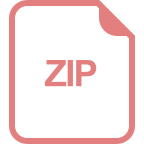
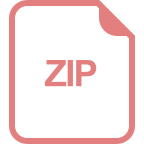
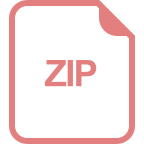
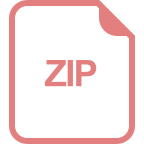
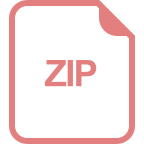
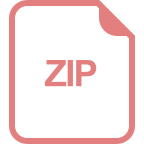