编写窗体应用程序,实现猜数字游戏。 在窗体中添加一个字段,保存系统所产生的谜底(随机数)。 在窗体的load事件中用Random对象产生一个100以内的整数作为谜底。 游戏这在窗体中输入所猜的数字,并单击按钮提交输入。 如果和谜底想等,则提示猜中,并询问游戏者是否再玩一次。 如果猜错,则向游戏者提示所猜数字是”大了“还是”小了“,并显示尝试次数。 PS:文本框中输入的文本是string类型,要把string转换为整型,convert.ToInt32等
时间: 2024-02-15 12:04:02 浏览: 130
好的,以下是 C# 猜数字游戏的代码示例:
```csharp
using System;
using System.Windows.Forms;
namespace GuessNumberGame
{
public partial class MainForm : Form
{
private int answer;
private int tries;
public MainForm()
{
InitializeComponent();
}
private void MainForm_Load(object sender, EventArgs e)
{
// 生成随机数作为答案
Random random = new Random();
answer = random.Next(1, 101);
tries = 0;
}
private void guessButton_Click(object sender, EventArgs e)
{
string inputText = inputTextBox.Text;
int guess = Convert.ToInt32(inputText);
if (guess == answer)
{
// 猜中了
triesLabel.Text = "你猜了 " + tries + " 次";
MessageBox.Show("恭喜你,猜中了!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
// 询问是否再玩一次
DialogResult result = MessageBox.Show("再玩一次?", "提示", MessageBoxButtons.YesNo, MessageBoxIcon.Question);
if (result == DialogResult.Yes)
{
// 重新开始游戏
answer = new Random().Next(1, 101);
tries = 0;
triesLabel.Text = "";
inputTextBox.Text = "";
}
else
{
Close();
}
}
else if (guess < answer)
{
// 猜小了
tries++;
triesLabel.Text = "你猜了 " + tries + " 次";
MessageBox.Show("猜小了!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
else
{
// 猜大了
tries++;
triesLabel.Text = "你猜了 " + tries + " 次";
MessageBox.Show("猜大了!", "提示", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
}
}
}
```
在这个示例中,我们在窗体的 `Load` 事件中生成一个随机数作为答案,并在 `Guess` 按钮的 `Click` 事件中判断用户输入的数字和答案是否相等。如果相等,则弹出提示框告知用户猜中了,并询问是否再玩一次;如果不相等,则根据用户输入的数字和答案的大小关系向用户提示猜大了还是猜小了,并记录尝试次数。另外,我们还需要将用户输入的字符串转换为整型,才能进行比较。
阅读全文
相关推荐
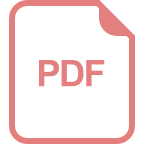
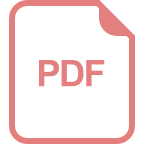
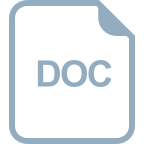
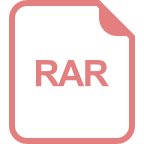
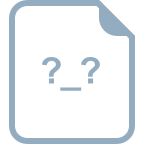
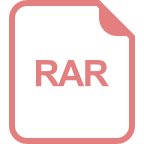
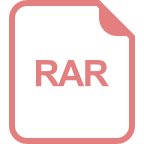
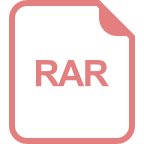
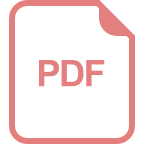
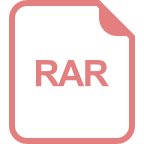
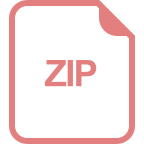
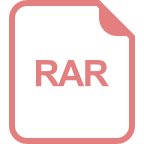
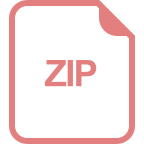
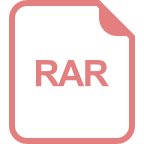