java中springboot框架如何对于数据库查询做异步处理请求
时间: 2024-03-26 20:35:14 浏览: 64
在Spring Boot中,可以使用异步处理来提高数据库查询的效率和性能。具体实现方法如下:
1. 在 pom.xml 文件中添加异步处理的依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-async</artifactId>
</dependency>
```
2. 在查询方法上添加 @Async 注解:
```
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Async
public Future<List<User>> findAllUsers() {
List<User> users = userRepository.findAll();
return new AsyncResult<>(users);
}
}
```
其中,@Async 注解表示该方法是异步处理,返回值类型为 Future,使用 AsyncResult 来封装异步处理方法的返回值。
3. 在调用查询方法的地方,使用 Future.get() 方法来获取异步处理方法的返回值:
```
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public List<User> findAllUsers() throws InterruptedException, ExecutionException {
Future<List<User>> future = userService.findAllUsers();
while (!future.isDone()) {
Thread.sleep(100);
}
return future.get();
}
}
```
其中,Future.get() 方法会阻塞主线程,直到异步处理方法执行完毕,返回异步处理的结果。
通过以上步骤,可以在 Spring Boot 中实现数据库查询的异步处理,提高查询效率和性能。
阅读全文
相关推荐
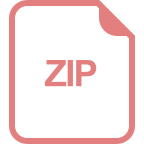
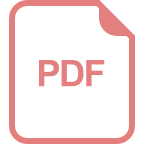
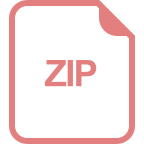
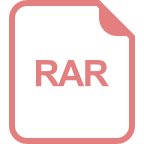
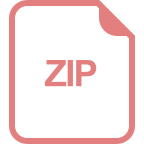
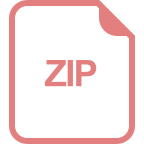
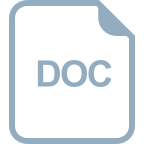
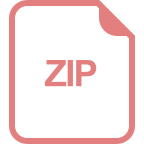
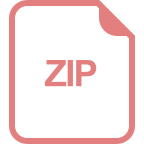
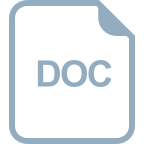
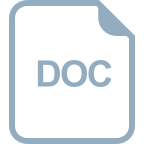






