用c++编写一个子函数实现月份全部输出,再编写一个子函数实现月份按字符串顺序排列,并将排列顺序结果进行输出
时间: 2024-02-05 22:11:02 浏览: 62
以下是使用C++编写的代码:
```c++
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
void outputAllMonths() {
string months[] = {"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"};
int numOfMonths = sizeof(months) / sizeof(months[0]);
for (int i = 0; i < numOfMonths; i++) {
cout << months[i] << endl;
}
}
void outputSortedMonths() {
string months[] = {"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"};
int numOfMonths = sizeof(months) / sizeof(months[0]);
sort(months, months + numOfMonths);
for (int i = 0; i < numOfMonths; i++) {
cout << months[i] << endl;
}
}
int main() {
cout << "All months:" << endl;
outputAllMonths();
cout << endl << "Sorted months:" << endl;
outputSortedMonths();
return 0;
}
```
首先我们定义了两个子函数,一个用于输出所有的月份,另一个用于按照字符串顺序排列并输出月份。在这两个子函数中,我们都使用了一个字符串数组来存储所有的月份,并利用 `sizeof` 运算符和数组元素类型的大小来计算出月份数组的长度。我们使用 `for` 循环遍历月份数组,输出数组中的每个元素。
对于按照字符串顺序排列并输出月份的函数,我们使用了 `std::sort` 算法来对月份数组进行排序。该算法会将数组中的元素按照字典序进行排序。最后,我们再次使用 `for` 循环遍历已排序的月份数组,并输出每个元素。
在 `main` 函数中,我们分别调用这两个子函数,并输出它们的结果。
阅读全文
相关推荐
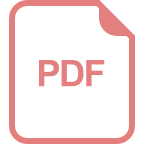




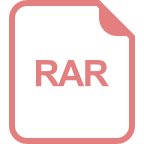
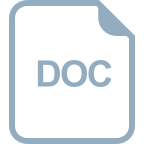
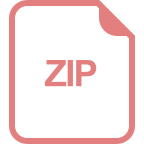
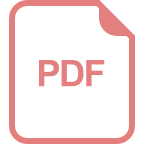
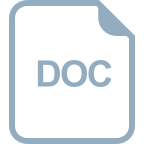
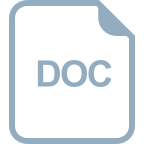
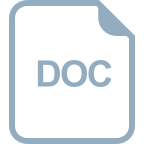
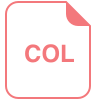
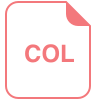
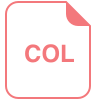
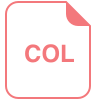
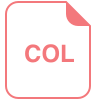