map循环 如何判断item是否包含某元素 代码
时间: 2024-01-31 22:04:36 浏览: 93
你可以使用JavaScript中的`includes`方法或者`indexOf`方法来判断`item`是否包含某元素,代码示例如下:
```
const items = ['元素1', '元素2', '元素3', '元素4']
items.map(item => {
if (item.includes('某元素')) {
console.log('包含某元素')
} else {
console.log('不包含某元素')
}
})
```
其中,`items`是一个包含多个元素的数组,`map`方法用于遍历数组中的每个元素,`includes`方法用于判断当前元素是否包含某个字符串(这里是'某元素'),如果包含则执行包含某元素的操作,否则执行不包含某元素的操作。`indexOf`方法的使用方式与`includes`类似,只需要将`includes`换成`indexOf`即可。
相关问题
遍历map,如果map中key包含_MAX,则放入另一个map
遍历Map(映射)并检查其中的键是否包含特定值,比如 "_MAX",可以通过迭代器或循环结构实现。这里是一个简单的示例代码片段,假设你正在使用像C++、Java或Python这样的语言:
```cpp
#include <iostream>
#include <unordered_map> // 使用C++的std::unordered_map
// 假设我们有一个map,例如 std::unordered_map<std::string, int>
std::unordered_map<std::string, int> original_map;
void process_map() {
std::unordered_map<std::string, int> new_map; // 新的map用来存放匹配的元素
for (const auto &entry : original_map) {
if (entry.first.find("_MAX") != std::string::npos) { // 如果找到"_MAX"
new_map[entry.first] = entry.second; // 把对应的值放到新map里
}
}
// 打印新map的内容
for (const auto &item : new_map) {
std::cout << "Key: " << item.first << ", Value: " << item.second << std::endl;
}
}
int main() {
// 初始化原始map...
process_map();
return 0;
}
```
或者,如果你是在Python中:
```python
original_map = {} # 使用字典替代map
def process_map():
new_map = {} # 创建新的字典
for key, value in original_map.items():
if '_MAX' in key:
new_map[key] = value
# 打印新字典的内容
for key, value in new_map.items():
print(f"Key: {key}, Value: {value}")
# 初始化原始字典...
process_map()
```
fatherclose(data) { this.totalData = [] let olddatalist = JSON.parse(JSON.stringify(this.dataList)) data.map((item) => { let ishave = false olddatalist.map((ele) => { if (ele.billNo == item.billNo) { ishave = true } }) if (!ishave) { this.dataList.push(item) } }) let fobj = {} this.totalFields.map((item) => { fobj[item] = this.getsum(item, this.dataList) }) let totalFields = [ 'totalBoxcount', 'totalCount', 'totalCubage', 'totalWeight', ] let footer = fobj this.footer = footer for (let key in footer) { this['totalData'].push({ key: key, value: footer[key], }) } this.pageOptions.pageTotal = this.dataList.length this.calcPageData() this.showaddDialog = false },
这段代码定义了一个名为 `fatherclose(data)` 的函数。让我逐步解释一下代码的逻辑:
1. 首先,将 `this.totalData` 数组置为空数组,用来存储计算后的总计数据。
2. 创建一个名为 `olddatalist` 的变量,通过深拷贝 `this.dataList` 数组的副本来初始化。
3. 使用 `.map((item) => { ... })` 方法遍历传入的 `data` 数组。
4. 在遍历过程中,使用一个布尔变量 `ishave` 来判断 `data` 中的元素在 `olddatalist` 中是否已存在。
5. 遍历 `olddatalist` 数组,并在每次迭代中检查当前元素的 `billNo` 属性是否与 `item` 的 `billNo` 属性相等。如果相等,则将 `ishave` 设置为 `true`。
6. 如果 `ishave` 为 `false`,表示 `item` 在 `olddatalist` 中不存在,则将 `item` 添加到 `this.dataList` 中。
7. 创建一个空对象 `fobj`,用来存储计算后的字段总和。
8. 使用 `.map((item) => { ... })` 方法遍历 `this.totalFields` 数组。
9. 在遍历过程中,将每个字段作为键,调用 `this.getsum(item, this.dataList)` 方法计算对应字段在 `this.dataList` 中的总和,并将结果存储在 `fobj[item]` 中。
10. 定义一个名为 `totalFields` 的数组,包含需要计算总和的字段名。
11. 将 `fobj` 赋值给 `this.footer`,用来更新表格的底部合计行。
12. 使用 `for...in` 循环遍历 `footer` 对象的键,并将键值对以 `{ key: key, value: footer[key] }` 的形式添加到 `this['totalData']` 数组中。
13. 更新分页组件的总记录数为 `this.dataList.length`,并重新计算分页数据。
14. 将 `this.showaddDialog` 设置为 `false`,关闭弹出框。
这段代码的主要作用是,将传入的数据添加到 `this.dataList` 中,并计算更新表格的底部合计行和分页数据。最后关闭弹出框。
阅读全文
相关推荐
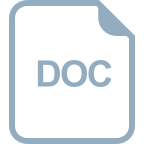
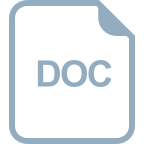
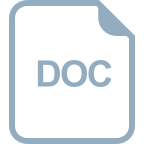
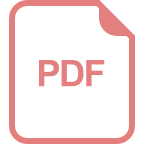
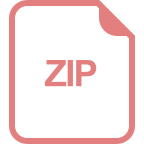
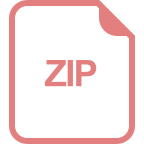
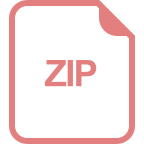
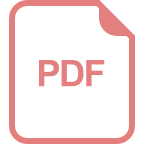
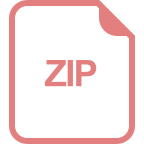
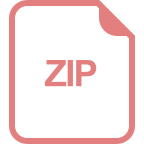
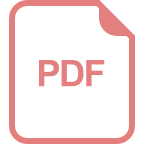
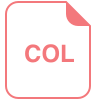
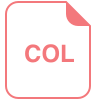


