使用Jupyter 构建可视化图形
时间: 2024-11-13 07:22:16 浏览: 3
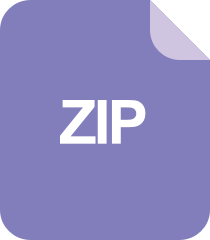
JupyterIPythonNotebook的算法可视化
在Jupyter Notebook中构建可视化图形通常涉及使用Python的数据科学库,如matplotlib、seaborn、plotly或更现代的库如Matplotlib库可以创建静态图表,如折线图、散点图、直方图等。Seaborn则提供了高级美观的统计图形,而Plotly支持交互式和动态图形。如果你需要更复杂的可视化,如网络图或地图,可以使用networkx(对于图)和geopandas(与地理数据结合)。
例如,以下是一个简单的例子,展示如何使用matplotlib创建一个条形图:
```python
import matplotlib.pyplot as plt
# 数据准备
labels = ['A', 'B', 'C', 'D']
values = [4, 8, 15, 16]
# 创建条形图
plt.bar(labels, values)
# 添加标题和标签
plt.title('Bar Chart Example')
plt.xlabel('Categories')
plt.ylabel('Values')
# 显示图形
plt.show()
```
对于交互式可视化,你可以使用`plotly.express`模块创建交互式的图表,如下所示:
```python
import plotly.express as px
df = px.data.tips() # 加载示例数据集
fig = px.bar(df, x='day', y='total_bill', title='Daily Total Bill')
fig.show()
```
阅读全文
相关推荐
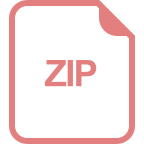
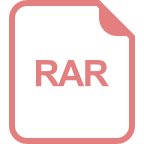
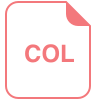
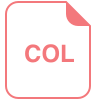
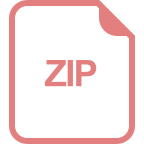
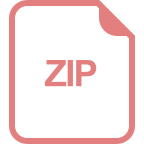
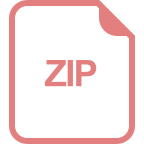
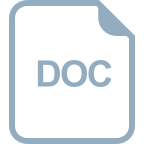
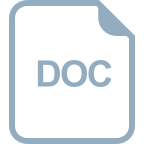
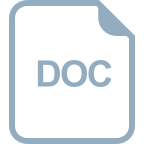
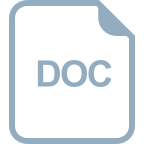
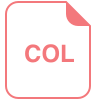
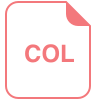
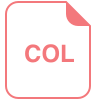
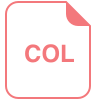
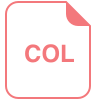
