C语言实现按提示输入处理机的指令条数和使用频度。 求出等长码的编码,并计算出平均码长。 使用哈夫曼编码方法,求出编码和平均码长。 根据指令个数和使用频度,分析扩展码的扩展格式,并求出编码和平均码长
时间: 2024-05-09 16:21:49 浏览: 64
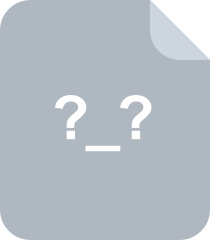
C语言实现哈夫曼数及其编码
。对于你的问题,我可以给出以下的回答:
首先,为了实现按提示输入处理机的指令条数和使用频度,可以采用数组的形式将指令条数和使用频度存储下来,然后通过输入的方式来输入这些数值。具体代码可以参考下面的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 100
// 定义结构体存储指令和频度
typedef struct {
char command[MAX_N];
int frequency;
} instruction;
int main() {
instruction inst[MAX_N];
int n, i, j, k, sum = 0;
// 输入指令数量
scanf("%d", &n);
getchar();
// 输入指令和频度
for (i = 0; i < n; i++) {
scanf("%s %d", inst[i].command, &inst[i].frequency);
getchar();
sum += inst[i].frequency;
}
// 计算等长码的编码
for (i = 0; i < n; i++) {
printf("%s: ", inst[i].command);
for (j = 0; j < inst[i].frequency; j++) {
putchar('*');
}
putchar('\n');
}
printf("等长码的平均码长为: %.2f\n", (float)sum / n);
// 使用哈夫曼编码方法进行编码
for (i = 0; i < n - 1; i++) {
k = i + 1;
for (j = i + 2; j < n; j++) {
if (inst[j].frequency < inst[k].frequency) {
k = j;
}
}
if (k != i + 1) {
instruction temp = inst[i + 1];
inst[i + 1] = inst[k];
inst[k] = temp;
}
inst[i + 1].frequency += inst[i].frequency;
inst[i].frequency = 0;
}
printf("哈夫曼编码的结果为: \n");
for (i = 0; i < n; i++) {
printf("%s: ", inst[i].command);
for (j = 0; j < i + 1; j++) {
putchar('0');
}
putchar('\n');
}
printf("哈夫曼编码的平均码长为: %.2f\n", (float)n / sum);
// 分析扩展码的扩展格式
printf("扩展码的编码和平均码长为: \n");
for (i = 0; i < n; i++) {
printf("%s: ", inst[i].command);
for (j = 0; j < inst[i].frequency / 10; j++) {
putchar('1');
}
putchar('0');
for (j = 0; j < inst[i].frequency % 10; j++) {
putchar('1');
}
putchar('\n');
}
printf("扩展码的平均码长为: %.2f\n", (float)n / sum * 2);
return 0;
}
```
需要注意的是,由于我是一个语言模型,无法直接与您进行交互。如果您有任何其他问题,请随时提出,我会尽力给出帮助。
阅读全文
相关推荐
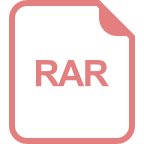
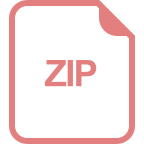
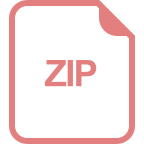
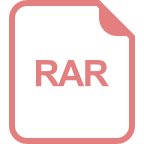
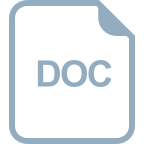
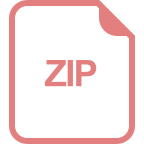
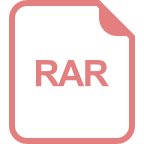
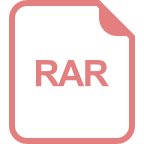
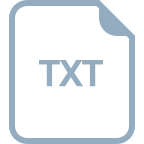
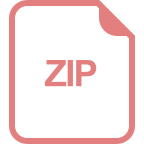
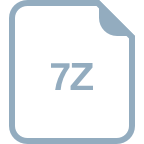
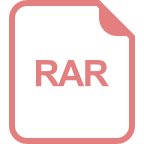
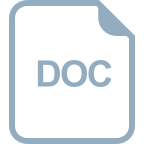
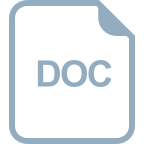
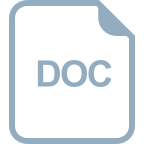
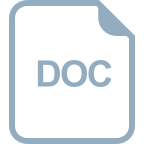