在一个游我动有各种各阵的经物,如约士,龙、狼,容等等。每个怪物都有生命力和攻击办。经物能够相互攻击,一个经物攻击分一个怪物时,放兵古者会受伤:同的,故攻击者会反击,使得攻击者也受伤 。但是一个经物反活的力量我弱,只是其攻击力的1/2。 ,超物士动政去,站我人攻法和实糖反法附都有相应的动作。例如,骑士攻古动作是挥舞宝到,所火龙的攻古动作是喷火:怪物受到攻击会 第叫和受份流血,如果受伤过面,生命力被城为0,则经物就会倒地 死去。 ,新对这个游戏,该如何编写程序,才能使得游戏版本开级、要增加新的经物时,原有的程序改动尽可能少呢? ,经物的攻击、反法和受伤等通过成员两数来实现。用c++编写龙和狼的类
时间: 2023-11-18 17:05:20 浏览: 141
好的,我理解了你的问题。针对你的问题,我可以提供一些思路和代码供你参考。
首先,我们可以设计一个基类 Creature(生物),包含一些所有经物都拥有的属性和方法,如生命值、攻击力、防御力、攻击方法、受伤方法等。然后,我们可以派生出 Dragon(龙)和 Wolf(狼)两个子类,分别实现它们特有的属性和方法。
下面是一个可能的实现:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
// 生物基类
class Creature {
public:
Creature(int hp, int attack) : m_hp(hp), m_attack(attack) {}
virtual ~Creature() {}
virtual void attack(Creature& other) {
cout << "Creature attacks " << typeid(other).name() << endl;
other.defend(m_attack);
}
virtual void defend(int attack) {
int damage = attack - m_defense;
if (damage < 0) damage = 0;
m_hp -= damage;
cout << "Creature takes " << damage << " damage" << endl;
if (m_hp <= 0) {
cout << "Creature is dead" << endl;
m_hp = 0;
}
}
protected:
int m_hp;
int m_attack;
int m_defense;
};
// 龙类
class Dragon : public Creature {
public:
Dragon(int hp, int attack) : Creature(hp, attack) {
m_defense = 5;
m_name = "Dragon";
}
virtual void attack(Creature& other) {
cout << "Dragon breathes fire on " << typeid(other).name() << endl;
other.defend(m_attack);
}
private:
string m_name;
};
// 狼类
class Wolf : public Creature {
public:
Wolf(int hp, int attack) : Creature(hp, attack) {
m_defense = 3;
m_name = "Wolf";
}
virtual void attack(Creature& other) {
cout << "Wolf bites " << typeid(other).name() << endl;
other.defend(m_attack);
}
private:
string m_name;
};
int main() {
srand(time(nullptr));
Dragon dragon(50, 10);
Wolf wolf(30, 8);
while (dragon.m_hp > 0 && wolf.m_hp > 0) {
int r = rand() % 2;
if (r == 0) {
dragon.attack(wolf);
} else {
wolf.attack(dragon);
}
cout << "Dragon HP: " << dragon.m_hp << endl;
cout << "Wolf HP: " << wolf.m_hp << endl;
cout << endl;
}
return 0;
}
```
在这个例子中,我们定义了一个 Creature 类作为基类,派生出了 Dragon 和 Wolf 两个子类。Dragon 类和 Wolf 类继承了 Creature 类的所有属性和方法,并分别实现了它们特有的攻击方法。
在主函数中,我们可以通过不断交替调用两个经物的攻击方法来模拟战斗,直到其中一个经物生命值为0为止。
这个程序可以很方便地扩展到支持更多的经物,只需要添加新的子类,并在主函数中创建相应的实例即可。
阅读全文
相关推荐
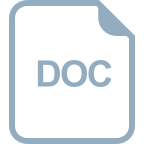
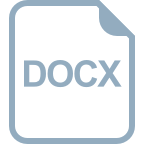
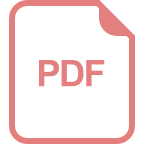
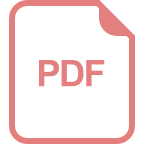
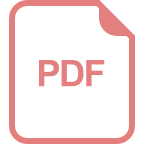
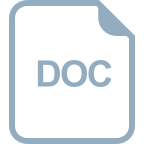
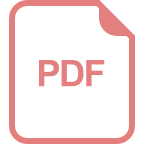
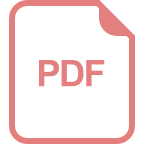
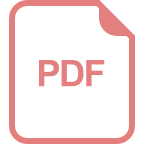
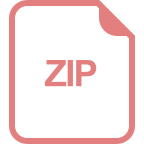
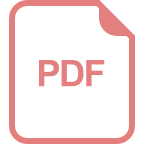
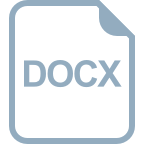
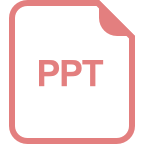
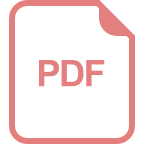
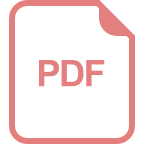
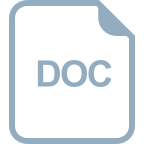
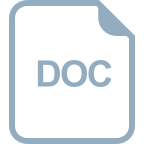
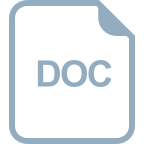
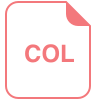
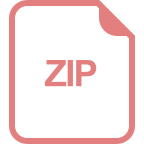