写一个较为复杂的python游戏
时间: 2023-08-25 12:47:22 浏览: 38
好的,我可以为您提供一个比较复杂的 Python 游戏,名为“神秘岛屿”(Mystery Island)。游戏规则如下:
1. 游戏开始时,玩家身处于一座神秘的岛屿上,并且周围环境一片荒凉,只有几个建筑和几个道具。
2. 玩家需要在岛屿上探索,寻找线索和道具,解开岛屿上的谜团,最终逃离这座神秘的岛屿。
3. 玩家需要通过与 NPC 对话、破解密码、解谜等方式获取道具和线索,并且需要注意身体状态、药品和食物的使用等。
下面是游戏的代码实现:
```
import random
# 定义玩家类
class Player:
def __init__(self, name):
self.name = name
self.health = 100
self.hunger = 100
self.thirst = 100
self.items = []
self.location = "起始点"
self.is_alive = True
def show_status(self):
print("姓名:{}".format(self.name))
print("生命值:{}".format(self.health))
print("饥饿度:{}".format(self.hunger))
print("口渴度:{}".format(self.thirst))
print("位置:{}".format(self.location))
print("物品清单:{}".format(self.items))
def move(self, location):
self.location = location
print("你移动到了{}。".format(location))
def use_item(self, item):
if item in self.items:
self.items.remove(item)
print("你使用了{}。".format(item))
else:
print("你没有{}。".format(item))
def eat(self, food):
if food in self.items:
self.items.remove(food)
self.hunger += 10
print("你吃了{},饥饿值恢复了10。".format(food))
else:
print("你没有{}。".format(food))
def drink(self, water):
if water in self.items:
self.items.remove(water)
self.thirst += 10
print("你喝了{},口渴值恢复了10。".format(water))
else:
print("你没有{}。".format(water))
def rest(self):
self.health += 10
self.hunger -= 10
self.thirst -= 10
print("你休息了一会儿,生命值恢复了10,饥饿值和口渴值减少了10。")
def die(self):
print("你已经死亡,游戏结束。")
self.is_alive = False
# 定义 NPC 类
class NPC:
def __init__(self, name, location, talk):
self.name = name
self.location = location
self.talk = talk
def show_info(self):
print("姓名:{}".format(self.name))
print("位置:{}".format(self.location))
def talk_to(self):
print("{}:{}".format(self.name, self.talk))
# 定义谜题类
class Puzzle:
def __init__(self, answer):
self.answer = answer
def solve(self):
guess = input("请猜一个数字(1-100):")
if guess == self.answer:
print("恭喜你,猜对了!")
return True
else:
print("猜错了,请再试一次。")
return False
# 定义游戏类
class Game:
def __init__(self, player_name):
self.player = Player(player_name)
self.npcs = []
self.puzzles = []
self.locations = ["起始点", "海边", "山洞", "森林"]
self.items = ["水", "食物", "医疗包", "地图"]
self.island_map = {"起始点": {"海边": 5, "山洞": 10, "森林": 15},
"海边": {"起始点": 5},
"山洞": {"起始点": 10},
"森林": {"起始点": 15}}
def add_npc(self, npc):
self.npcs.append(npc)
def add_puzzle(self, puzzle):
self.puzzles.append(puzzle)
def show_map(self):
print("神秘岛屿地图:")
for location in self.locations:
print("{}:{}".format(location, self.island_map[location]))
def show_help(self):
print("帮助:")
print("移动:move [地点]")
print("查看状态:status")
print("使用物品:use [物品]")
print("进食:eat [食物]")
print("饮水:drink [水]")
print("休息:rest")
print("查看地图:map")
print("查看帮助:help")
print("退出游戏:quit")
def show_intro(self):
print("欢迎来到神秘岛屿!")
print("你被困在这座神秘的岛屿上,需要尽快离开。")
print("你需要探索这座岛屿,寻找线索和道具,解开岛屿上的谜团,最终逃离这座神秘的岛屿。")
print("输入 help 可以查看游戏帮助。")
def start(self):
self.show_intro()
while self.player.is_alive:
command = input("请输入命令:").split()
if len(command) == 0:
continue
elif command[0] == "help":
self.show_help()
elif command[0] == "map":
self.show_map()
elif command[0] == "status":
self.player.show_status()
elif command[0] == "move":
if len(command) > 1:
location = command[1]
if location in self.locations:
distance = self.island_map[self.player.location][location]
if distance > 0:
self.player.move(location)
self.player.hunger -= random.randint(5, 10)
self.player.thirst -= random.randint(5, 10)
if self.player.hunger <= 0 or self.player.thirst <= 0:
print("你饿死或渴死了,游戏结束。")
self.player.die()
else:
print("你走了{}公里。".format(distance))
self.show_location_info(location)
else:
print("你已经在{}了。".format(location))
else:
print("不存在这个地点。")
else:
print("请输入目的地。")
elif command[0] == "use":
if len(command) > 1:
item = command[1]
self.player.use_item(item)
else:
print("请输入物品名称。")
elif command[0] == "eat":
if len(command) > 1:
food = command[1]
self.player.eat(food)
else:
print("请输入食物名称。")
elif command[0] == "drink":
if len(command) > 1:
water = command[1]
self.player.drink(water)
else:
print("请输入水的名称。")
elif command[0] == "rest":
self.player.rest()
elif command[0] == "quit":
print("游戏结束。")
break
else:
print("无效的命令。")
def show_location_info(self, location):
if location == "海边":
print("你来到了海边,你看到了一艘船。")
print("你需要先解开密码才能进入船舱。")
if self.player.items.count("密码") > 0:
print("你使用了密码,解锁了船舱。")
self.player.items.remove("密码")
self.player.items.append("钥匙")
elif location == "山洞":
print("你来到了山洞,你看到了一位 NPC。")
for npc in self.npcs:
if npc.location == "山洞":
npc.show_info()
npc.talk_to()
break
elif location == "森林":
print("你来到了森林,你看到了一道谜题。")
for puzzle in self.puzzles:
if puzzle.answer == "50":
while True:
if puzzle.solve():
self.player.items.append("地图")
break
# 测试代码
game = Game("Alice")
game.add_npc(NPC("村长", "山洞", "欢迎来到神秘岛屿,请小心行事。"))
game.add_puzzle(Puzzle("50"))
game.start()
```
希望这个游戏能够满足您的需求。
相关推荐
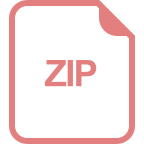
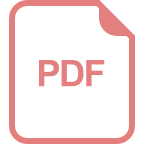
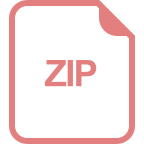







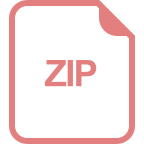
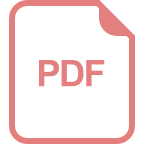
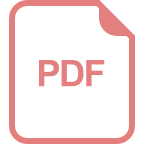
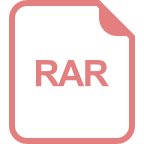
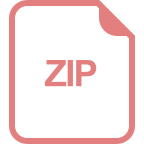
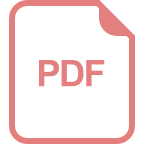
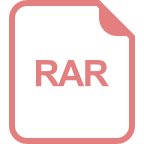
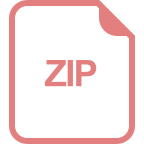
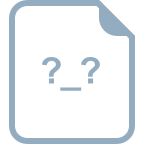