Java Geometry接口
时间: 2023-06-01 16:01:45 浏览: 77
Java Geometry是一个接口,定义了一组基本的几何操作和数据结构,用于处理平面和空间中的图形和形状。该接口提供了一些常用的几何计算方法,如计算线段长度、计算两点之间的距离、计算点是否在多边形内等。
Java Geometry接口包含以下方法:
1. length():计算线段长度。
2. distance(Point2D p):计算当前点与指定点之间的距离。
3. contains(Point2D p):判断当前图形是否包含指定的点。
4. intersects(Rectangle2D r):判断当前图形是否与指定矩形相交。
5. intersects(Line2D l):判断当前图形是否与指定线段相交。
6. getBounds():返回当前图形的边界矩形。
7. getCenter():返回当前图形的中心点。
8. getPerimeter():返回当前图形的周长。
9. getArea():返回当前图形的面积。
Java Geometry接口可以用于实现各种图形,如点、线段、多边形、圆形等。它还可以用于计算图形之间的相交、包含关系等。
相关问题
java编写为柱体的底面设计一个接口 Geometry,包含计算面积的方法 getArea(),和计算周长方法 getC()
好的,这是一个关于Java编程的问题。为柱体的底面设计一个接口Geometry,包含计算面积的方法getArea()和计算周长方法getC(),可以这样实现:
public interface Geometry {
double getArea();
double getC();
}
然后,我们可以创建一个类来实现这个接口,比如:
public class Cylinder implements Geometry {
private double radius;
private double height;
public Cylinder(double radius, double height) {
this.radius = radius;
this.height = height;
}
@Override
public double getArea() {
return 2 * Math.PI * radius * radius + 2 * Math.PI * radius * height;
}
@Override
public double getC() {
return 2 * Math.PI * radius;
}
}
这个类实现了Geometry接口,并提供了具体的计算方法。我们可以在其他地方使用这个类来计算柱体的面积和周长。
mybatis几何geometry类型转换
MyBatis 中对于几何(Geometry)类型的转换,可以通过使用 TypeHandler 来实现。TypeHandler 是 MyBatis 提供的一个接口,用于将 Java 对象和数据库中的数据进行转换。
对于几何类型的转换,你可以自定义一个实现了 TypeHandler 接口的类,然后在 MyBatis 的配置文件中进行注册。
以下是一个示例的 TypeHandler 实现:
```java
import org.apache.ibatis.type.BaseTypeHandler;
import org.apache.ibatis.type.JdbcType;
import java.sql.CallableStatement;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import com.vividsolutions.jts.geom.Geometry;
import com.vividsolutions.jts.io.WKTReader;
public class GeometryTypeHandler extends BaseTypeHandler<Geometry> {
@Override
public void setNonNullParameter(PreparedStatement ps, int i, Geometry parameter, JdbcType jdbcType) throws SQLException {
ps.setString(i, parameter.toText());
}
@Override
public Geometry getNullableResult(ResultSet rs, String columnName) throws SQLException {
String geometryString = rs.getString(columnName);
return parseGeometry(geometryString);
}
@Override
public Geometry getNullableResult(ResultSet rs, int columnIndex) throws SQLException {
String geometryString = rs.getString(columnIndex);
return parseGeometry(geometryString);
}
@Override
public Geometry getNullableResult(CallableStatement cs, int columnIndex) throws SQLException {
String geometryString = cs.getString(columnIndex);
return parseGeometry(geometryString);
}
private Geometry parseGeometry(String geometryString) {
if (geometryString != null) {
WKTReader reader = new WKTReader();
try {
return reader.read(geometryString);
} catch (Exception e) {
// Handle exception
}
}
return null;
}
}
```
在以上的示例代码中,我们使用了 JTS Topology Suite(JTS)来处理几何类型。在 `setNonNullParameter` 方法中,我们将 Geometry 对象转换为 WKT(Well-Known Text)格式的字符串,并设置到 PreparedStatement 中。在 `getNullableResult` 方法中,我们从 ResultSet 或 CallableStatement 中取出字符串,并将其解析为 Geometry 对象。
接下来,在 MyBatis 的配置文件中注册这个 TypeHandler:
```xml
<typeHandlers>
<typeHandler handler="com.example.GeometryTypeHandler"/>
</typeHandlers>
```
需要注意的是,你需要根据你自己的数据库和几何类型库选择合适的依赖和实现方式。以上示例代码仅供参考,具体实现可能会有所差异。
相关推荐
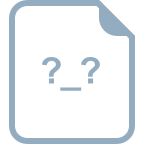
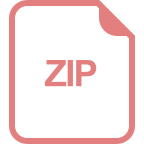












