怎样编写一个程序能读取二进制文件并将其编码为视频帧然后再编写一个解码程序,将接收到的视频帧解码回二进制数据并验证其完整性。
时间: 2024-09-24 16:15:38 浏览: 47
要编写这样的程序,你需要了解视频编码的基本原理和技术,比如H.264或MPEG等常见的压缩标准。以下是基本步骤:
**编码程序(假设使用Python with OpenCV库)**:
1. **导入所需库**:
```python
import cv2
import numpy as np
from PIL import Image
```
2. **读取二进制文件**:
```python
with open('in.bin', 'rb') as binary_file:
data = binary_file.read()
```
3. **创建一个VideoWriter对象**:
```python
fourcc = cv2.VideoWriter_fourcc(*'mp4v') # H.264编码
height, width, _ = len(data) // (width * height), width, 3 # 假设原始数据是图像像素,计算尺寸
video_writer = cv2.VideoWriter('encoded.mp4', fourcc, fps=30, frameSize=(width, height))
```
4. **将二进制数据转换为视频帧**:
```python
for i in range(0, len(data), width * height * 3): # 每次循环处理一行像素
img_data = data[i:i+width*height*3]
img = np.frombuffer(img_data, dtype=np.uint8).reshape((height, width, 3)) # 将字节转为RGB图像
video_writer.write(img)
```
5. **关闭 VideoWriter 对象**:
```python
video_writer.release()
```
**解码程序(同样使用OpenCV)**:
1. **打开编码后的视频文件**:
```python
cap = cv2.VideoCapture('encoded.mp4')
```
2. **获取视频帧大小**:
```python
_, frame = cap.read()
width, height = frame.shape[1], frame.shape[0]
```
3. **循环读取帧并将解码后的数据写入二进制文件**:
```python
while True:
ret, frame = cap.read()
if not ret:
break
raw_data = frame.tobytes() # 将帧转换为二进制
with open('decoded.bin', 'ab') as output_file:
output_file.write(raw_data)
```
4. **验证完整性**:
```python
decoded_data = open('decoded.bin', 'rb').read()
assert len(decoded_data) == len(data), "Decoded data size does not match original"
correct_bits = 0
for i in range(min(len(decoded_data), len(data))):
if decoded_data[i] == data[i]:
correct_bits += 1
accuracy = correct_bits / len(decoded_data)
print(f"Decoding accuracy: {accuracy * 100}%")
```
请注意,实际实现可能需要考虑更多因素,如视频编码效率、错误处理和性能优化。同时,这个例子假设了输入文件是图像数据,如果实际情况不同,可能需要调整处理方式。
阅读全文
相关推荐






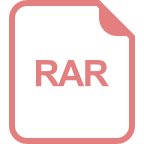











