smartfile类
时间: 2025-01-01 16:20:16 浏览: 11
### SmartFile 类的概述
SmartFile 类的设计旨在简化文件处理过程,提供更便捷的操作方式。此类封装了常见的文件读写功能,并提供了额外的功能增强,如错误处理机制和流式操作支持。
#### 功能特性
- 文件创建与打开
- 支持多种编码格式
- 提供同步与异步两种模式下的读取和写入方法
- 自动管理资源释放
- 错误恢复能力
#### 定义与实现
以下是 Python 中 `SmartFile` 的基本定义:
```python
class SmartFile:
"""A smart file handler class that simplifies common file operations."""
def __init__(self, filename, mode='r', encoding=None):
self.filename = filename
self.mode = mode
self.encoding = encoding
self.file_handle = None
def open(self):
try:
self.file_handle = open(
self.filename,
self.mode,
encoding=self.encoding
)
except IOError as e:
print(f"Failed to open file: {e}")
def read(self):
if not hasattr(self, 'file_handle') or self.file_handle is None:
raise ValueError("File has not been opened yet.")
content = ''
try:
with self.file_handle as f:
content = f.read()
finally:
return content
def write(self, data):
if not hasattr(self, 'file_handle') or self.file_handle is None:
raise ValueError("File has not been opened yet.")
try:
with self.file_handle as f:
f.write(data)
except Exception as ex:
print(f"Error writing to file: {ex}")
def close(self):
if self.file_handle and not self.file_handle.closed:
self.file_handle.close()
def __enter__(self):
self.open()
return self
def __exit__(self, exc_type, exc_val, exc_tb):
self.close()
```
此段代码展示了 `SmartFile` 类的核心结构及其主要成员函数[^1]。
#### 使用示例
下面是一个简单的例子,展示如何利用 `SmartFile` 进行文件读写操作:
```python
with SmartFile('example.txt', 'w+', encoding='utf8') as sf:
sf.write("这是一个测试字符串。\n")
text = sf.read()
print(text)
# 输出应为:“这是一个测试字符串。”
```
阅读全文
相关推荐

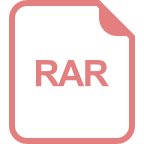
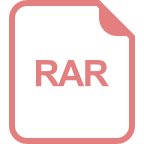

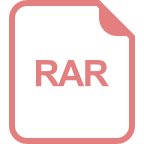
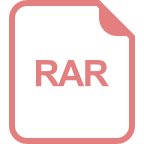

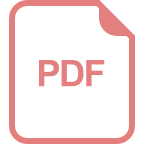
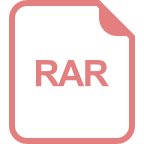


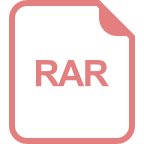
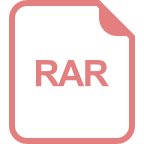
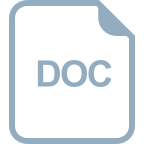




