Implement the knn_classifier function with Python,
时间: 2024-05-05 16:15:57 浏览: 135
Here's an implementation of the k-nearest neighbors (KNN) classifier in Python:
```
import numpy as np
def knn_classifier(X_train, y_train, X_test, k):
"""
K-nearest neighbors classifier
Parameters:
X_train (numpy.ndarray): Training data features
y_train (numpy.ndarray): Training data labels
X_test (numpy.ndarray): Test data features
k (int): Number of nearest neighbors to consider
Returns:
y_pred (numpy.ndarray): Predicted labels for test data
"""
# Calculate distances between test data and training data
dists = np.sqrt(np.sum((X_train - X_test[:, np.newaxis])**2, axis=2))
# Get indices of k nearest neighbors for each test data point
knn_indices = np.argsort(dists, axis=1)[:, :k]
# Get labels of k nearest neighbors
knn_labels = y_train[knn_indices]
# Predict labels based on majority vote
y_pred = np.apply_along_axis(lambda x: np.bincount(x).argmax(), axis=1, arr=knn_labels)
return y_pred
```
This function takes in the training data features (`X_train`) and labels (`y_train`), test data features (`X_test`), and the number of nearest neighbors to consider (`k`). It first calculates the distances between each test data point and each training data point using the Euclidean distance metric. It then finds the indices of the k nearest neighbors for each test data point, and gets the corresponding labels. Finally, it predicts the label of each test data point based on the majority vote of its k nearest neighbors.
Note that this implementation assumes that the input data is in the form of numpy arrays. If your data is in a different format, you may need to modify the function accordingly. Also, this implementation uses the `np.apply_along_axis()` function to apply a function to each row of a 2D array. This can be slower than using a loop, but is more concise and often easier to read. If performance is a concern, you may want to consider using a loop instead.
阅读全文
相关推荐
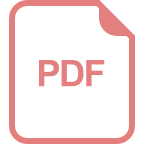
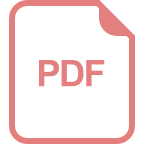
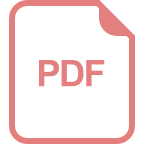
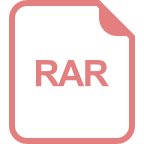
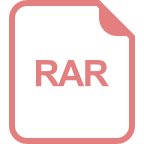
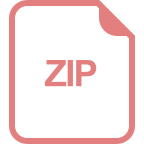
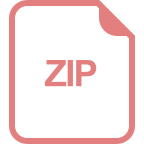
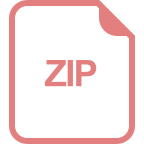
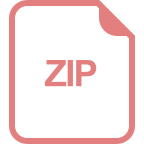
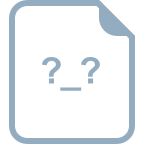
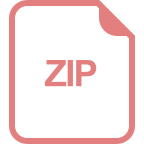
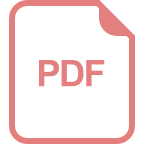
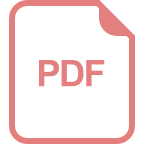
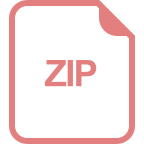
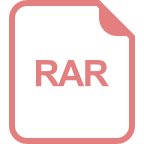
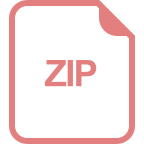
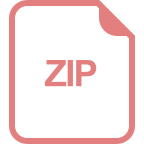
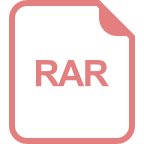