怎么导入PySimpleGUI库
时间: 2024-06-19 15:03:28 浏览: 143
要导入PySimpleGUI库,您需要先安装Python环境。然后可以通过以下步骤来导入PySimpleGUI库:
1. 打开命令提示符或终端。
2. 在命令提示符或终端中输入以下命令:`pip install PySimpleGUI`。
3. 等待安装完成后,您可以在Python中导入PySimpleGUI库,方法如下:
```python
import PySimpleGUI as sg
```
这样就可以使用PySimpleGUI库了。如果您使用的是Anaconda等Python发行版,也可以通过Anaconda Navigator或其他方式安装PySimpleGUI库。
相关问题
用Python(推荐导入PySimpleGUI库)写一个面积计算器,要求能计算矩形面积,三角形面积,圆形面积,并且界面不同
好的,以下是一个基于PySimpleGUI的面积计算器的代码:
```python
import math
import PySimpleGUI as sg
# 定义矩形面积计算函数
def calc_rect_area(length, width):
return length * width
# 定义三角形面积计算函数
def calc_tri_area(base, height):
return 0.5 * base * height
# 定义圆形面积计算函数
def calc_circle_area(radius):
return math.pi * radius ** 2
# 定义主窗口布局
layout = [
[sg.Text('计算矩形面积', font=('Arial', 16))],
[sg.Text('长度:'), sg.InputText(size=(10,1), key='rect_length')],
[sg.Text('宽度:'), sg.InputText(size=(10,1), key='rect_width')],
[sg.Button('计算', key='rect_calc_btn')],
[sg.Text('矩形面积为:', key='rect_output')],
[sg.HorizontalSeparator()],
[sg.Text('计算三角形面积', font=('Arial', 16))],
[sg.Text('底边长:'), sg.InputText(size=(10,1), key='tri_base')],
[sg.Text('高:'), sg.InputText(size=(10,1), key='tri_height')],
[sg.Button('计算', key='tri_calc_btn')],
[sg.Text('三角形面积为:', key='tri_output')],
[sg.HorizontalSeparator()],
[sg.Text('计算圆形面积', font=('Arial', 16))],
[sg.Text('半径:'), sg.InputText(size=(10,1), key='circle_radius')],
[sg.Button('计算', key='circle_calc_btn')],
[sg.Text('圆形面积为:', key='circle_output')]
]
# 创建主窗口
window = sg.Window('面积计算器', layout)
# 主循环
while True:
event, values = window.read()
if event == sg.WIN_CLOSED:
break
elif event == 'rect_calc_btn':
try:
length = float(values['rect_length'])
width = float(values['rect_width'])
area = calc_rect_area(length, width)
window['rect_output'].update('矩形面积为:{}'.format(area))
except ValueError:
window['rect_output'].update('请输入数字!')
elif event == 'tri_calc_btn':
try:
base = float(values['tri_base'])
height = float(values['tri_height'])
area = calc_tri_area(base, height)
window['tri_output'].update('三角形面积为:{}'.format(area))
except ValueError:
window['tri_output'].update('请输入数字!')
elif event == 'circle_calc_btn':
try:
radius = float(values['circle_radius'])
area = calc_circle_area(radius)
window['circle_output'].update('圆形面积为:{}'.format(area))
except ValueError:
window['circle_output'].update('请输入数字!')
# 关闭窗口
window.close()
```
运行以上代码后,将会弹出一个带有三个不同计算器的窗口,每个计算器的界面都不同,如下图所示:

用Python(推荐导入PySimpleGUI库)写一个面积计算器,能在主界面选择计算哪种图形的面积,然后跳转计算矩形面积,三角形面积,圆形面积的界面
好的,以下是一个使用PySimpleGUI库编写的面积计算器示例:
```python
import math
import PySimpleGUI as sg
# 矩形面积计算页面布局
rect_layout = [
[sg.Text('请输入矩形的长和宽')],
[sg.Text('长:', size=(10, 1)), sg.InputText(key='rect_length')],
[sg.Text('宽:', size=(10, 1)), sg.InputText(key='rect_width')],
[sg.Button('计算', key='rect_calc'), sg.Button('返回', key='rect_back')]
]
# 三角形面积计算页面布局
tri_layout = [
[sg.Text('请输入三角形的底和高')],
[sg.Text('底:', size=(10, 1)), sg.InputText(key='tri_base')],
[sg.Text('高:', size=(10, 1)), sg.InputText(key='tri_height')],
[sg.Button('计算', key='tri_calc'), sg.Button('返回', key='tri_back')]
]
# 圆形面积计算页面布局
circle_layout = [
[sg.Text('请输入圆的半径')],
[sg.Text('半径:', size=(10, 1)), sg.InputText(key='circle_radius')],
[sg.Button('计算', key='circle_calc'), sg.Button('返回', key='circle_back')]
]
# 主页面布局
main_layout = [
[sg.Text('请选择要计算面积的图形')],
[sg.Button('矩形', key='rect_btn', size=(10, 2)), sg.Button('三角形', key='tri_btn', size=(10, 2)), sg.Button('圆形', key='circle_btn', size=(10, 2))],
[sg.Output(size=(30, 10))]
]
# 创建窗口
window = sg.Window('面积计算器', main_layout)
# 主循环
while True:
event, values = window.read()
if event == sg.WINDOW_CLOSED:
break
# 点击矩形按钮,跳转到矩形面积计算页面
if event == 'rect_btn':
rect_window = sg.Window('矩形面积计算', rect_layout)
while True:
rect_event, rect_values = rect_window.read()
if rect_event == sg.WINDOW_CLOSED or rect_event == 'rect_back':
rect_window.close()
break
if rect_event == 'rect_calc':
length = float(rect_values['rect_length'])
width = float(rect_values['rect_width'])
area = length * width
print(f'矩形面积为:{area:.2f}')
# 点击三角形按钮,跳转到三角形面积计算页面
elif event == 'tri_btn':
tri_window = sg.Window('三角形面积计算', tri_layout)
while True:
tri_event, tri_values = tri_window.read()
if tri_event == sg.WINDOW_CLOSED or tri_event == 'tri_back':
tri_window.close()
break
if tri_event == 'tri_calc':
base = float(tri_values['tri_base'])
height = float(tri_values['tri_height'])
area = 0.5 * base * height
print(f'三角形面积为:{area:.2f}')
# 点击圆形按钮,跳转到圆形面积计算页面
elif event == 'circle_btn':
circle_window = sg.Window('圆形面积计算', circle_layout)
while True:
circle_event, circle_values = circle_window.read()
if circle_event == sg.WINDOW_CLOSED or circle_event == 'circle_back':
circle_window.close()
break
if circle_event == 'circle_calc':
radius = float(circle_values['circle_radius'])
area = math.pi * radius ** 2
print(f'圆形面积为:{area:.2f}')
window.close()
```
运行上述代码,会弹出一个主界面,可以选择要计算的图形。点击相应的按钮后,会跳转到对应的计算页面,输入参数后点击计算按钮,即可计算出相应图形的面积。
阅读全文
相关推荐
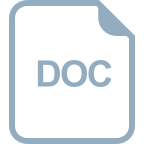
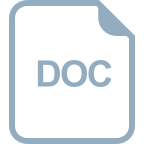
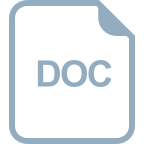









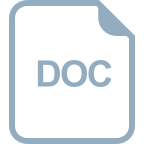
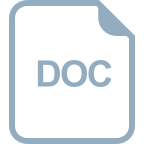
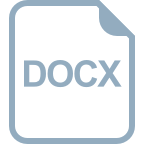