用c++写用面向对象的方法编写通讯录管理系统(包括:姓名,电话号码,QQ,Email,籍贯等信息 )。 增加(注意:姓名、电话号码、QQ是唯一的。不能有重复记录存在。) 删除(可以按照姓名、电话号码、QQ进行操作) 修改(可以按照姓名、电话号码、QQ进行操作) 查询(可以按照姓名、电话号码、QQ进行操作)
时间: 2023-07-10 22:10:03 浏览: 95
好的,这是一个比较典型的面向对象编程的示例。首先,我们需要定义一个 Contact 类来存储通讯录中每个人的信息,同时实现增删改查的功能。下面是一个简单的实现示例:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Contact {
public:
Contact(){}
Contact(string name, string phone, string qq, string email, string addr) :
m_name(name), m_phone(phone), m_qq(qq), m_email(email), m_addr(addr) {}
string getName() const { return m_name; }
string getPhone() const { return m_phone; }
string getQQ() const { return m_qq; }
string getEmail() const { return m_email; }
string getAddr() const { return m_addr; }
void setName(string name) { m_name = name; }
void setPhone(string phone) { m_phone = phone; }
void setQQ(string qq) { m_qq = qq; }
void setEmail(string email) { m_email = email; }
void setAddr(string addr) { m_addr = addr; }
private:
string m_name;
string m_phone;
string m_qq;
string m_email;
string m_addr;
};
class ContactManager {
public:
void addContact(const Contact& contact) {
if (findContact(contact.getName(), contact.getPhone(), contact.getQQ()) == nullptr) {
m_contacts.push_back(contact);
cout << "Add contact successfully!" << endl;
}
else {
cout << "Add contact failed! The contact already exists." << endl;
}
}
void removeContact(const string& name, const string& phone, const string& qq) {
for (auto it = m_contacts.begin(); it != m_contacts.end(); ++it) {
if (it->getName() == name || it->getPhone() == phone || it->getQQ() == qq) {
m_contacts.erase(it);
cout << "Remove contact successfully!" << endl;
return;
}
}
cout << "Remove contact failed! The contact does not exist." << endl;
}
void modifyContact(const Contact& contact, const string& name, const string& phone, const string& qq) {
auto p = findContact(name, phone, qq);
if (p != nullptr) {
*p = contact;
cout << "Modify contact successfully!" << endl;
}
else {
cout << "Modify contact failed! The contact does not exist." << endl;
}
}
Contact* findContact(const string& name, const string& phone, const string& qq) {
for (auto it = m_contacts.begin(); it != m_contacts.end(); ++it) {
if (it->getName() == name || it->getPhone() == phone || it->getQQ() == qq) {
return &(*it);
}
}
return nullptr;
}
void listContacts() const {
cout << "Name\tPhone\tQQ\tEmail\tAddress" << endl;
for (const auto& contact : m_contacts) {
cout << contact.getName() << "\t" << contact.getPhone() << "\t" << contact.getQQ()
<< "\t" << contact.getEmail() << "\t" << contact.getAddr() << endl;
}
}
private:
vector<Contact> m_contacts;
};
int main() {
ContactManager mgr;
Contact c1("Alice", "123456789", "12345", "alice@example.com", "Beijing");
mgr.addContact(c1);
Contact c2("Bob", "987654321", "54321", "bob@example.com", "Shanghai");
mgr.addContact(c2);
mgr.listContacts();
Contact c3("Charlie", "135792468", "24680", "charlie@example.com", "Guangzhou");
mgr.addContact(c3);
mgr.listContacts();
mgr.removeContact("Bob", "", "");
mgr.listContacts();
Contact c4("Daniel", "246813579", "13579", "daniel@example.com", "Hangzhou");
mgr.modifyContact(c4, "Alice", "", "12345");
mgr.listContacts();
return 0;
}
```
在这个实现中,我们用 Contact 类来存储每个人的信息,其中包括姓名、电话、QQ、邮箱和地址等信息,同时还实现了 get 和 set 方法来获取和修改每个属性的值。
ContactManager 类是用来管理通讯录的,其中包括 addContact、removeContact、modifyContact 和 findContact 等方法。其中,addContact 方法用来添加新的联系人,需要判断姓名、电话和 QQ 是否已经存在;removeContact 方法用来删除联系人,可以按照姓名、电话和 QQ 进行删除;modifyContact 方法用来修改联系人信息,同样可以按照姓名、电话和 QQ 进行修改;findContact 方法用来查找联系人信息,同样可以按照姓名、电话和 QQ 进行查找。
最后,在 main 函数中,我们可以通过 ContactManager 对象来进行增删改查等操作,然后输出通讯录中的所有联系人信息。
阅读全文
相关推荐
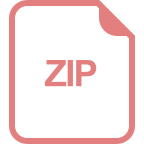
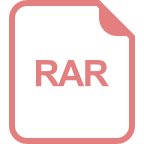
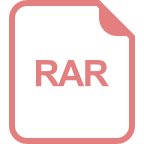
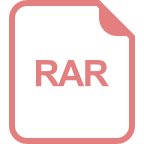
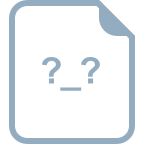
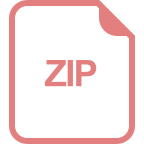
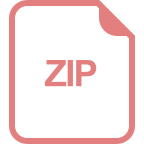
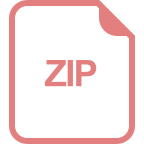
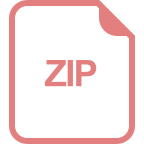
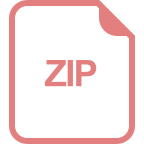
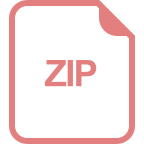
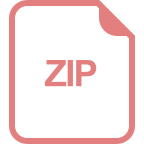
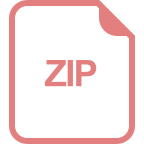
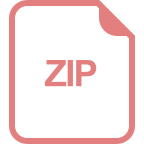
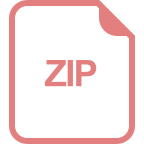