用Verilog实现一个ieee1588协议
时间: 2024-02-21 20:58:19 浏览: 167
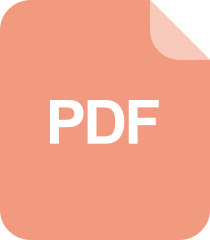
IIC通信协议的Verilog实现
好的,您的问题是如何用Verilog实现一个IEEE 1588协议。IEEE 1588是一种用于精确时间同步的协议,通常用于工业自动化和通信系统。
实现IEEE 1588需要在FPGA或ASIC中实现一个精确的时钟同步模块,该模块可以通过网络将时钟同步到微秒级别。以下是一个简单的Verilog代码示例,用于实现IEEE 1588协议:
```
module ieee1588 (
input clk,
input rst,
input ptp_start,
input ptp_stop,
input ptp_reset,
input ptp_event,
input [47:0] ptp_timestamp,
output [47:0] ptp_adjustment
);
// Declare internal signals
reg [31:0] count;
reg run;
reg sync;
reg adjust;
wire event;
// Implement the PTP state machine
always @(posedge clk or posedge rst) begin
if (rst) begin
count <= 0;
run <= 0;
sync <= 0;
adjust <= 0;
end else begin
case ({run, sync, adjust})
3'b000: begin // IDLE state
run <= ptp_start;
end
3'b001: begin // WAIT state
if (ptp_event) begin
count <= ptp_timestamp;
sync <= 1;
end else if (ptp_stop) begin
run <= 0;
end
end
3'b010: begin // SYNC state
if (count > 0) begin
count <= count - 1;
end else begin
sync <= 0;
adjust <= 1;
end
end
3'b011: begin // ADJUST state
if (ptp_reset) begin
adjust <= 0;
run <= 0;
end
end
endcase
end
end
// Implement the PTP event detection logic
assign event = (run && sync && !adjust);
// Implement the PTP adjustment logic
always @(posedge clk or posedge rst) begin
if (rst) begin
ptp_adjustment <= 0;
end else if (adjust) begin
ptp_adjustment <= {48{event}};
end
end
endmodule
```
上述代码实现了一个简单的状态机,该状态机实现了PTP协议的关键功能。在实现过程中,需要注意以下几点:
1. 时钟同步模块需要通过网络接收和发送PTP消息。
2. 在实现状态机时,需要注意状态的转移条件和时序约束。
3. 在实现时钟调整逻辑时,需要注意时钟精度和时钟漂移的影响。
希望这个简单的Verilog代码示例可以帮助您实现IEEE 1588协议。
阅读全文
相关推荐
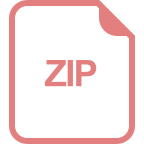
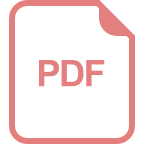
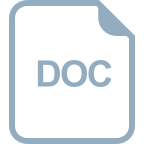
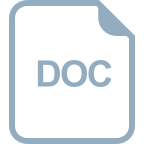
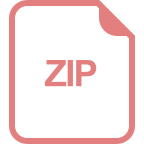
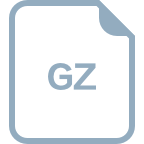
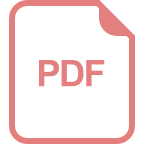
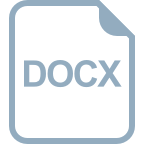
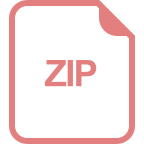
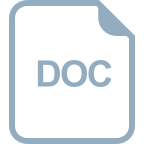
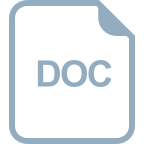
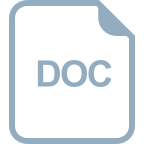

