spring security Springboot使用代码实现
时间: 2023-10-28 12:05:27 浏览: 90
1. 引入依赖
在pom.xml文件中添加spring-boot-starter-security依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 配置安全策略
在application.properties中配置安全策略:
```properties
# 关闭基本认证
security.basic.enabled=false
# 允许所有用户访问所有端点
security.require-ssl=false
security.enable-csrf=false
```
3. 创建用户服务
创建一个实现UserDetailsService接口的用户服务,用于加载用户信息:
```java
@Service
public class UserDetailsServiceImpl implements UserDetailsService {
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
// 这里可以从数据库或其他地方加载用户信息
if ("admin".equals(username)) {
return new User("admin", "{noop}admin", Collections.singletonList(new SimpleGrantedAuthority("ROLE_ADMIN")));
} else if ("user".equals(username)) {
return new User("user", "{noop}user", Collections.singletonList(new SimpleGrantedAuthority("ROLE_USER")));
} else {
throw new UsernameNotFoundException("用户不存在");
}
}
}
```
4. 配置安全过滤器
在配置类中添加@EnableWebSecurity和@Configuration注解,并继承WebSecurityConfigurerAdapter,然后配置安全过滤器:
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private UserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN") // /admin/** 接口需要 ADMIN 角色
.antMatchers("/user/**").hasRole("USER") // /user/** 接口需要 USER 角色
.anyRequest().authenticated() // 其他接口需要认证
.and()
.formLogin()
.loginProcessingUrl("/login") // 登录接口路径
.defaultSuccessURL("/index") // 登录成功后跳转到 /index 接口
.failureUrl("/login?error") // 登录失败跳转到 /login?error 接口
.permitAll() // 允许所有用户访问登录接口
.and()
.logout()
.logoutUrl("/logout") // 退出登录接口路径
.logoutSuccessUrl("/login?logout") // 退出登录成功后跳转到 /login?logout 接口
.permitAll(); // 允许所有用户访问退出登录接口
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return NoOpPasswordEncoder.getInstance();
}
}
```
5. 创建控制器
创建控制器,用于测试接口访问权限:
```java
@RestController
public class TestController {
@GetMapping("/admin/hello")
public String adminHello() {
return "Hello, Admin!";
}
@GetMapping("/user/hello")
public String userHello() {
return "Hello, User!";
}
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
```
6. 测试接口
启动应用,然后使用管理员账户(admin/admin)和普通用户账户(user/user)访问不同的接口:
- http://localhost:8080/admin/hello 只有管理员能访问,需要管理员账户登录。
- http://localhost:8080/user/hello 只有普通用户能访问,需要普通用户账户登录。
- http://localhost:8080/hello 所有用户都能访问,不需要登录。
阅读全文
相关推荐
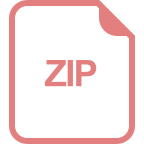
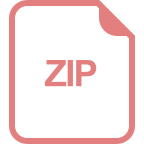
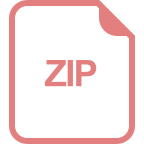
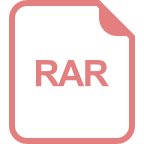
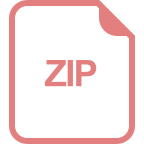
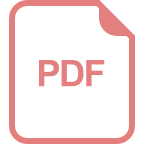
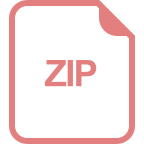
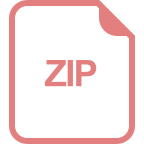
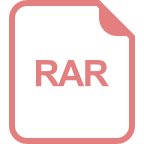
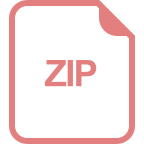
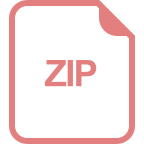
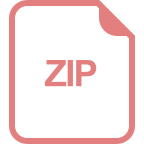
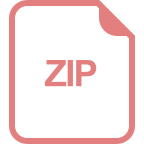
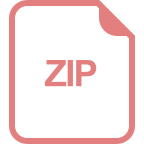
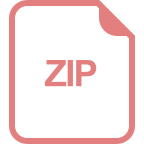