android studio实现收货地址管理的代码
时间: 2023-06-30 19:05:56 浏览: 331
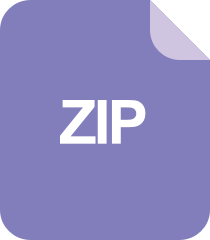
Android程序中的收货地址管理实现

以下是Android Studio实现收货地址管理的代码示例:
1. 创建收货地址实体类(Address.java)
```java
public class Address {
private String name; // 收货人姓名
private String phone; // 收货人电话
private String address; // 收货地址
public Address(String name, String phone, String address) {
this.name = name;
this.phone = phone;
this.address = address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
```
2. 创建收货地址列表适配器(AddressAdapter.java)
```java
public class AddressAdapter extends RecyclerView.Adapter<AddressAdapter.ViewHolder> {
private List<Address> addressList;
static class ViewHolder extends RecyclerView.ViewHolder {
TextView nameView;
TextView phoneView;
TextView addressView;
public ViewHolder(View itemView) {
super(itemView);
nameView = itemView.findViewById(R.id.name);
phoneView = itemView.findViewById(R.id.phone);
addressView = itemView.findViewById(R.id.address);
}
}
public AddressAdapter(List<Address> addressList) {
this.addressList = addressList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.address_item, parent, false);
ViewHolder holder = new ViewHolder(view);
return holder;
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
Address address = addressList.get(position);
holder.nameView.setText(address.getName());
holder.phoneView.setText(address.getPhone());
holder.addressView.setText(address.getAddress());
}
@Override
public int getItemCount() {
return addressList.size();
}
}
```
3. 创建收货地址编辑页面(AddressEditActivity.java)
```java
public class AddressEditActivity extends AppCompatActivity {
private EditText nameEdit;
private EditText phoneEdit;
private EditText addressEdit;
private boolean isEdit; // 是否是编辑模式
private Address address; // 编辑模式下的地址
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_address_edit);
nameEdit = findViewById(R.id.name_edit);
phoneEdit = findViewById(R.id.phone_edit);
addressEdit = findViewById(R.id.address_edit);
Intent intent = getIntent();
if (intent.hasExtra("address")) {
isEdit = true;
address = (Address) intent.getSerializableExtra("address");
nameEdit.setText(address.getName());
phoneEdit.setText(address.getPhone());
addressEdit.setText(address.getAddress());
} else {
isEdit = false;
}
Button saveButton = findViewById(R.id.save_button);
saveButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = nameEdit.getText().toString();
String phone = phoneEdit.getText().toString();
String addressStr = addressEdit.getText().toString();
if (TextUtils.isEmpty(name)) {
Toast.makeText(AddressEditActivity.this, "请填写收货人姓名", Toast.LENGTH_SHORT).show();
return;
}
if (TextUtils.isEmpty(phone)) {
Toast.makeText(AddressEditActivity.this, "请填写收货人电话", Toast.LENGTH_SHORT).show();
return;
}
if (TextUtils.isEmpty(addressStr)) {
Toast.makeText(AddressEditActivity.this, "请填写收货地址", Toast.LENGTH_SHORT).show();
return;
}
if (isEdit) {
address.setName(name);
address.setPhone(phone);
address.setAddress(addressStr);
Toast.makeText(AddressEditActivity.this, "修改成功", Toast.LENGTH_SHORT).show();
} else {
Address newAddress = new Address(name, phone, addressStr);
MainActivity.addressList.add(newAddress);
Toast.makeText(AddressEditActivity.this, "添加成功", Toast.LENGTH_SHORT).show();
}
finish();
}
});
}
}
```
4. 创建收货地址列表页面(MainActivity.java)
```java
public class MainActivity extends AppCompatActivity {
public static List<Address> addressList = new ArrayList<Address>();
private AddressAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RecyclerView recyclerView = findViewById(R.id.recycler_view);
LinearLayoutManager layoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
adapter = new AddressAdapter(addressList);
recyclerView.setAdapter(adapter);
Button addButton = findViewById(R.id.add_button);
addButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this, AddressEditActivity.class);
startActivity(intent);
}
});
}
@Override
protected void onResume() {
super.onResume();
adapter.notifyDataSetChanged();
}
}
```
5. 创建收货地址列表项布局文件(address_item.xml)
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<TextView
android:id="@+id/name"
android:textSize="18sp"
android:textStyle="bold"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/phone"
android:textSize="16sp"
android:layout_marginTop="8dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<TextView
android:id="@+id/address"
android:textSize="16sp"
android:layout_marginTop="8dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
6. 创建收货地址编辑页面布局文件(activity_address_edit.xml)
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp">
<TextView
android:text="收货人姓名"
android:textSize="18sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/name_edit"
android:hint="请输入收货人姓名"
android:textSize="16sp"
android:layout_marginTop="8dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:text="收货人电话"
android:textSize="18sp"
android:layout_marginTop="16dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/phone_edit"
android:hint="请输入收货人电话"
android:textSize="16sp"
android:layout_marginTop="8dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:text="收货地址"
android:textSize="18sp"
android:layout_marginTop="16dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<EditText
android:id="@+id/address_edit"
android:hint="请输入收货地址"
android:textSize="16sp"
android:layout_marginTop="8dp"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<Button
android:id="@+id/save_button"
android:text="保存"
android:textSize="16sp"
android:layout_marginTop="16dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
```
以上是实现收货地址管理的代码示例,你可以根据自己的需求进行修改和完善。
阅读全文
相关推荐
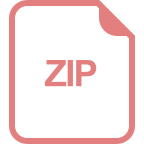
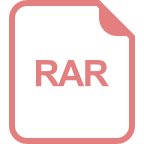

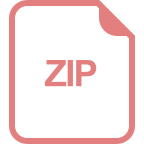
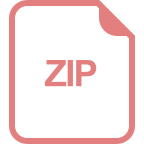
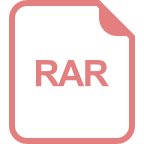
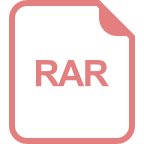
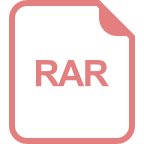
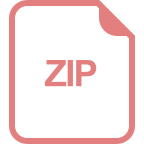
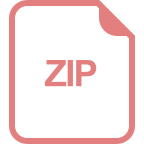
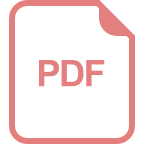
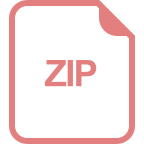
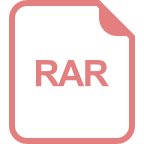
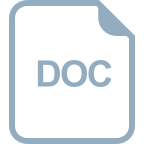
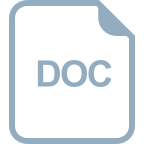
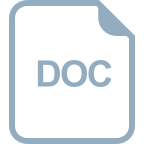

