图书管理系统借阅管理c语言
时间: 2023-12-30 11:24:07 浏览: 72
根据提供的引用内容,以下是一个简单的C语言图书管理系统的借阅管理功能的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
#define MAX_BORROWERS 100
typedef struct {
int bookId;
char title[100];
char author[100];
int isBorrowed;
} Book;
typedef struct {
int borrowerId;
char name[100];
int borrowedBooks[MAX_BOOKS];
int numBorrowedBooks;
} Borrower;
Book books[MAX_BOOKS];
Borrower borrowers[MAX_BORROWERS];
int numBooks = 0;
int numBorrowers = 0;
void addBook() {
if (numBooks >= MAX_BOOKS) {
printf("The library is full.\n");
return;
}
Book newBook;
printf("Enter book ID: ");
scanf("%d", &newBook.bookId);
printf("Enter book title: ");
scanf("%s", newBook.title);
printf("Enter book author: ");
scanf("%s", newBook.author);
newBook.isBorrowed = 0;
books[numBooks] = newBook;
numBooks++;
printf("Book added successfully.\n");
}
void borrowBook() {
int bookId, borrowerId;
printf("Enter book ID: ");
scanf("%d", &bookId);
printf("Enter borrower ID: ");
scanf("%d", &borrowerId);
Book *book = NULL;
for (int i = 0; i < numBooks; i++) {
if (books[i].bookId == bookId) {
book = &books[i];
break;
}
}
Borrower *borrower = NULL;
for (int i = 0; i < numBorrowers; i++) {
if (borrowers[i].borrowerId == borrowerId) {
borrower = &borrowers[i];
break;
}
}
if (book == NULL || borrower == NULL) {
printf("Invalid book ID or borrower ID.\n");
return;
}
if (book->isBorrowed) {
printf("The book is already borrowed.\n");
return;
}
if (borrower->numBorrowedBooks >= MAX_BOOKS) {
printf("The borrower has reached the maximum number of borrowed books.\n");
return;
}
book->isBorrowed = 1;
borrower->borrowedBooks[borrower->numBorrowedBooks] = bookId;
borrower->numBorrowedBooks++;
printf("Book borrowed successfully.\n");
}
void returnBook() {
int bookId, borrowerId;
printf("Enter book ID: ");
scanf("%d", &bookId);
printf("Enter borrower ID: ");
scanf("%d", &borrowerId);
Book *book = NULL;
for (int i = 0; i < numBooks; i++) {
if (books[i].bookId == bookId) {
book = &books[i];
break;
}
}
Borrower *borrower = NULL;
for (int i = 0; i < numBorrowers; i++) {
if (borrowers[i].borrowerId == borrowerId) {
borrower = &borrowers[i];
break;
}
}
if (book == NULL || borrower == NULL) {
printf("Invalid book ID or borrower ID.\n");
return;
}
if (!book->isBorrowed) {
printf("The book is not borrowed.\n");
return;
}
int found = 0;
for (int i = 0; i < borrower->numBorrowedBooks; i++) {
if (borrower->borrowedBooks[i] == bookId) {
borrower->borrowedBooks[i] = borrower->borrowedBooks[borrower->numBorrowedBooks - 1];
borrower->numBorrowedBooks--;
found = 1;
break;
}
}
if (!found) {
printf("The borrower did not borrow this book.\n");
return;
}
book->isBorrowed = 0;
printf("Book returned successfully.\n");
}
void displayBooks() {
printf("Books in the library:\n");
for (int i = 0; i < numBooks; i++) {
printf("Book ID: %d\n", books[i].bookId);
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Status: %s\n", books[i].isBorrowed ? "Borrowed" : "Available");
printf("\n");
}
}
void displayBorrowers() {
printf("Borrowers:\n");
for (int i = 0; i < numBorrowers; i++) {
printf("Borrower ID: %d\n", borrowers[i].borrowerId);
printf("Name: %s\n", borrowers[i].name);
printf("Borrowed books:\n");
for (int j = 0; j < borrowers[i].numBorrowedBooks; j++) {
printf("- Book ID: %d\n", borrowers[i].borrowedBooks[j]);
}
printf("\n");
}
}
int main() {
int choice;
do {
printf("1. Add book\n");
printf("2. Borrow book\n");
printf("3. Return book\n");
printf("4. Display books\n");
printf("5. Display borrowers\n");
printf("0. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addBook();
break;
case 2:
borrowBook();
break;
case 3:
returnBook();
break;
case 4:
displayBooks();
break;
case 5:
displayBorrowers();
break;
case 0:
printf("Exiting...\n");
break;
default:
printf("Invalid choice.\n");
break;
}
printf("\n");
} while (choice != 0);
return 0;
}
```
阅读全文
相关推荐


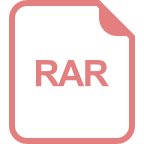









