vue let time=’06:00:00‘ let time1 = ’08:00:00‘ time和time1相对比
时间: 2024-11-12 07:44:04 浏览: 4
在Vue.js中,`let time='06:00:00'` 和 `let time1='08:00:00'` 是字符串类型的变量,存储了时间格式的文本数据。如果你想比较两个时间点,你需要先将它们转换成JavaScript Date 对象来进行处理。
你可以使用内置的Date构造函数或者第三方库如 moment.js 或者 date-fns 来实现这个转换。例如:
```javascript
// 假设你有一个函数可以解析并比较时间
function compareTimes(timeStr, timeStr1) {
const time = new Date(timeStr);
const time1 = new Date(timeStr1);
if (time < time1) {
return -1;
} else if (time > time1) {
return 1;
} else {
return 0; // 时间相等
}
}
const result = compareTimes(time, time1);
```
在这个例子中,`compareTimes` 函数会返回一个数字表示两个时间的顺序:-1表示第一个时间早于第二个,1表示第一个时间晚于第二个,0表示两者相等。
相关问题
vue let list = [{id: "5564018360057856" time: "2024-09-09T07:23:19.436Z"}] let id = '123' 判断数组里id是否含有这个id的内容
在JavaScript中,如果你想判断一个对象数组中是否含有具有特定`id`的对象,你可以使用`Array.prototype.find`方法或者`Array.prototype.some`方法。这里是一个简单的例子,展示如何实现这个功能:
```javascript
let list = [{id: "5564018360057856", time: "2024-09-09T07:23:19.436Z"}];
let id = '123';
// 使用find方法查找是否有对象的id属性与给定id相等
let found = list.find(item => item.id === id);
// 如果找到了,found变量会是一个对象,否则为undefined
if (found) {
console.log('找到了ID为', id, '的对象');
} else {
console.log('没有找到ID为', id, '的对象');
}
// 或者使用some方法检查是否有对象的id属性与给定id相等
let containsId = list.some(item => item.id === id);
console.log('数组中包含ID为', id, '的对象:', containsId);
```
这段代码首先定义了一个对象数组`list`和一个要查找的`id`值。使用`find`方法可以获取到第一个匹配的对象,如果找到的话。如果没有找到,`find`方法会返回`undefined`。另外一种方法是使用`some`方法,它会返回一个布尔值,告诉你数组中是否至少有一个元素满足条件。
vue遍历数组times2: [ { time: '8:00',click: false }, { time: '9:00',click: true }, {time: '10:00', click: false }, { time: '11:00',click: false }, { time: '12:00', click: true }, { time: '13:00', click: true }, { time: '14:00', click: true }, { time: '15:00', click: true }, { time: '16:00', click: false }, { time: '8:00',click: false }, { time: '9:00',click: true }, { time: '10:00', click: false }, { time: '11:00', click: false }, { time: '12:00', click: true }, { time: '13:00', click: true }, { time: '14:00', click: true }, { time: '15:00', click: true }, { time: '16:00', click: false }, ]实现生成多个方格,click的值表示该方格是否可以选中,可选为蓝色,不可选为灰色,从鼠标按下触发的方格开始,拖动选中多个方格,到鼠标弹起触发的方格结束,只可选择一段且连续的方格。选中的方格颜色为红色,
可以使用 v-for 指令遍历数组,根据 click 的值来设置方格的颜色。在鼠标按下事件中记录当前选中的方格,鼠标移动时根据当前鼠标所在的方格和按下时记录的方格来确定选中的方格范围,再根据范围设置选中方格的颜色为红色。在鼠标弹起事件中取消选中状态。具体实现可以参考以下代码:
```html
<template>
<div>
<div v-for="(item, index) in times2" :key="index"
:class="['time-cell', item.click ? 'clickable' : '']"
:style="{ backgroundColor: item.color }"
@mousedown="startSelect(index)"
@mousemove="select(index)"
@mouseup="endSelect">
{{ item.time }}
</div>
</div>
</template>
<script>
export default {
data() {
return {
times2: [
{ time: '8:00', click: false, color: '' },
{ time: '9:00', click: true, color: '' },
{ time: '10:00', click: false, color: '' },
{ time: '11:00', click: false, color: '' },
{ time: '12:00', click: true, color: '' },
{ time: '13:00', click: true, color: '' },
{ time: '14:00', click: true, color: '' },
{ time: '15:00', click: true, color: '' },
{ time: '16:00', click: false, color: '' },
{ time: '8:00', click: false, color: '' },
{ time: '9:00', click: true, color: '' },
{ time: '10:00', click: false, color: '' },
{ time: '11:00', click: false, color: '' },
{ time: '12:00', click: true, color: '' },
{ time: '13:00', click: true, color: '' },
{ time: '14:00', click: true, color: '' },
{ time: '15:00', click: true, color: '' },
{ time: '16:00', click: false, color: '' },
],
selecting: false,
start: -1,
end: -1,
};
},
methods: {
startSelect(index) {
if (this.times2[index].click) {
this.selecting = true;
this.start = index;
this.end = index;
this.setColor(index, 'red');
}
},
select(index) {
if (this.selecting) {
if (this.times2[index].click) {
if (index < this.start) {
this.setColor(this.end, '');
this.setColor(index, 'red');
this.end = this.start;
this.start = index;
} else if (index > this.end) {
this.setColor(this.start, '');
this.setColor(index, 'red');
this.start = this.end;
this.end = index;
} else {
this.setColor(this.start, '');
this.setColor(index, 'red');
this.start = index;
}
} else {
this.endSelect();
}
}
},
endSelect() {
if (this.selecting) {
this.selecting = false;
for (let i = this.start; i <= this.end; i++) {
this.setColor(i, this.times2[i].click ? 'blue' : '');
}
this.start = -1;
this.end = -1;
}
},
setColor(index, color) {
this.$set(this.times2[index], 'color', color);
},
},
};
</script>
<style scoped>
.time-cell {
width: 50px;
height: 50px;
border: 1px solid black;
display: inline-block;
text-align: center;
line-height: 50px;
cursor: pointer;
}
.clickable {
background-color: blue;
}
.red {
background-color: red !important;
}
.gray {
background-color: gray !important;
}
</style>
```
阅读全文
相关推荐
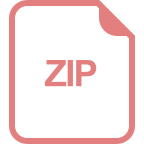
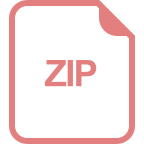


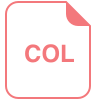










