springboot整合spring+mybatis
时间: 2023-09-02 08:11:04 浏览: 44
要在Spring Boot中整合Spring和MyBatis,你可以按照以下步骤进行配置:
1. 添加Spring Boot和MyBatis的依赖到`pom.xml`文件中:
```xml
<dependencies>
<!-- Spring Boot -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- MyBatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
</dependency>
<!-- 其他依赖... -->
</dependencies>
```
2. 创建一个Spring配置类,可以使用`@Configuration`注解标记,并在该类中定义需要配置的Spring Bean,例如数据源和事务管理器:
```java
@Configuration
public class SpringConfig {
// 数据源配置
@Bean
@ConfigurationProperties(prefix = "spring.datasource")
public DataSource dataSource() {
return DataSourceBuilder.create().build();
}
// MyBatis SqlSessionFactory配置
@Bean
public SqlSessionFactory sqlSessionFactory(DataSource dataSource) throws Exception {
SqlSessionFactoryBean factoryBean = new SqlSessionFactoryBean();
factoryBean.setDataSource(dataSource);
return factoryBean.getObject();
}
// 事务管理器配置
@Bean
public PlatformTransactionManager transactionManager(DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
}
```
3. 创建一个Mapper接口,使用MyBatis的注解来定义SQL映射和查询方法。例如:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM users")
List<User> getAllUsers();
}
```
4. 在Spring Boot的主类上添加`@MapperScan`注解,指定Mapper接口所在的包:
```java
@SpringBootApplication
@MapperScan("com.example.mapper")
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
5. 在业务代码中使用`@Autowired`注解将需要的Mapper接口注入进来并使用。
这样就完成了Spring Boot整合Spring和MyBatis的配置。你可以根据具体需求进行配置和扩展。希望对你有帮助!如果还有其他问题,请随时提问。
相关推荐
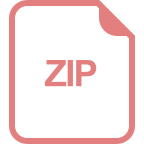
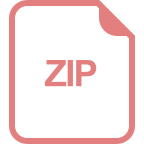
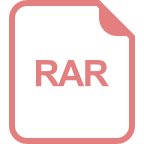














