vue 父子组件之间怎么传递参数
时间: 2024-11-03 13:21:06 浏览: 32
Vue.js 中父子组件间的参数传递有多种方式:
1. **props** (属性): 最常用的方式,父组件通过`props`向下传递数据给子组件。父组件通过标签属性传递值,并定义好相应的`props`类型和默认值。子组件通过`this.$ props`访问这些数据。
```html
<!-- 父组件 -->
<template>
<child-component :message="parentMessage"/>
</template>
<script>
export default {
data() {
return { parentMessage: 'Hello from parent' };
}
};
</script>
```
```js
// 子组件 ChildComponent.vue
<template>
<p>{{ message }}</p>
</template>
<script>
import { Prop, Component } from 'vue';
@Component
export default {
props: {
// 定义接收的 prop 名称、类型以及是否允许空值
message: { type: String, required: true },
},
};
</script>
```
2. **事件(Event Emitters)**: 父组件通过触发自定义事件并监听这个事件,在子组件中处理事件携带的数据。
```html
<!-- 父组件 -->
<template>
<button @click="passData('fromParent')">Pass Data</button>
<child-component @receiveData="handleReceivedData"></child-component>
</template>
<script>
export default {
methods: {
passData(data) {
this.$emit('receiveData', data);
},
handleReceivedData(data) {
console.log('Received:', data);
}
}
};
</script>
```
3. **Vuex** (状态管理库): 当数据需要在应用的多个部分共享时,可以考虑使用 Vuex。父子组件通过actions和mutations间接通信。
```javascript
// store.js 或者 vuex 中
export const actions = {
sendData({ commit }, payload) {
commit('SET_DATA', payload);
}
};
// 父组件 dispatch
dispatch('sendData', 'parentData');
// mutations.js
const mutations = {
SET_DATA(state, data) {
state.parentData = data;
}
};
```
阅读全文
相关推荐
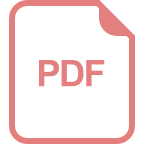
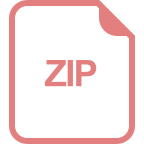
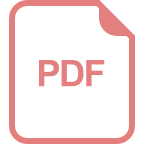
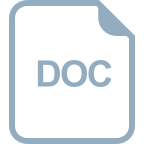




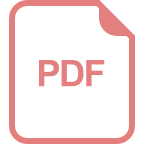
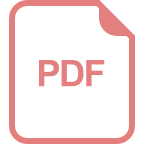
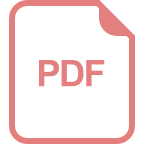






