用c++写:输入随机字符串包含英文字母、数字以及特殊字符等ASCI范围内字符· 输出为字符串中的数字字符,并按数字大小从小到大排序,重复数字不合并 示例:输入 3d50J,Aa3 输出:0335简写程序·,不要使用容器
时间: 2024-10-13 15:18:35 浏览: 37
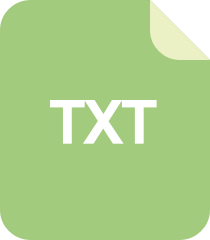
统计字符串中字母和数字个数
要在C++中实现上述功能,我们可以创建一个自定义的迭代器来遍历输入字符串并过滤出数字字符。这里是一个简单的解决方案,它不依赖于标准库容器:
```cpp
#include <iostream>
#include <string>
#include <algorithm>
class NumberIterator {
private:
std::string str;
std::string current;
char lastChar = '\0';
int num = 0;
public:
explicit NumberIterator(const std::string& input)
: str(input), current(str.begin()) {}
bool operator==(const NumberIterator& other) const {
return current == str.end() && other.current == other.str.end();
}
bool operator!=(const NumberIterator& other) const {
return !(*this == other);
}
void next() {
while (current != str.end()) {
char c = *current++;
if ('0' <= c && c <= '9') {
if (c != lastChar) {
num = c - '0';
lastChar = c;
} else {
num *= 10;
num += c - '0';
}
} else {
break;
}
}
}
int getNumber() const {
return num;
}
};
void printSortedNumbers(const std::string& input) {
NumberIterator it(input);
while (!it == end()) {
std::cout << it.getNumber();
it.next();
}
std::cout << "\n";
}
int main() {
std::string input;
std::cout << "请输入字符串: ";
std::getline(std::cin, input);
printSortedNumbers(input);
return 0;
}
```
在这个程序中,我们创建了一个名为`NumberIterator`的迭代器,它遍历输入字符串,每次遇到数字字符就更新当前数字并存储上次遇到的数字字符。当遇到非数字字符或遍历结束时,输出当前的数字。
运行这个程序后,它会提示用户输入一个字符串,然后按照题目要求输出字符串中的所有数字字符,按照从小到大的顺序排列且重复数字不合并。
阅读全文
相关推荐
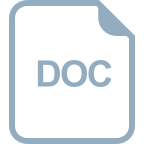
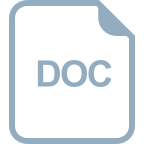




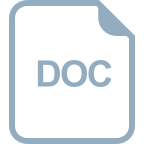
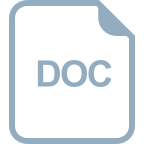
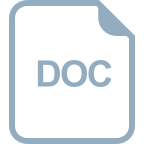







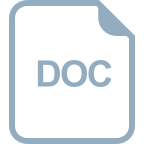
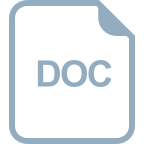