数据结构用顺序表实现两个递增集合的合并,用c语言程序表示
时间: 2024-09-15 22:09:15 浏览: 43
数据结构中,我们可以使用顺序表(数组)来实现两个递增集合的合并。以下是简单的C语言程序示例:
```c
#include <stdio.h>
// 结构体定义用于存储元素和指针
typedef struct {
int data;
struct Node* next;
} Node;
// 创建新的节点
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 合并两个递增集合的函数
void mergeSortedLists(Node** list1, Node** list2) {
// 初始化临时指针
Node* temp1 = *list1, *temp2 = *list2;
// 如果第一个列表为空,则直接复制第二个列表
if (!temp1) {
*list1 = temp2;
return;
}
// 如果第二个列表为空,或者第一个列表当前元素小于第二个列表
while (temp2 && temp1->data <= temp2->data) {
// 将第二个列表的元素添加到第一个列表的末尾
temp1->next = temp2;
temp1 = temp1->next;
temp2 = temp2->next;
}
// 将第一个列表剩余部分连接回去
if (temp1) {
temp1->next = *list2;
} else {
*list1 = temp2;
}
}
// 测试用法
int main() {
Node* list1 = createNode(1);
list1->next = createNode(4);
list1->next->next = createNode(7);
Node* list2 = createNode(2);
list2->next = createNode(5);
list2->next->next = createNode(6);
// 合并两个列表
mergeSortedLists(&list1, &list2);
// 打印合并后的结果
Node* current = list1;
while (current) {
printf("%d ", current->data);
current = current->next;
}
return 0;
}
阅读全文
相关推荐
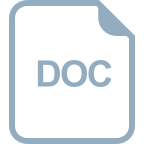
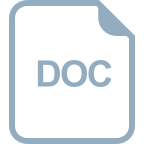
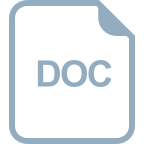
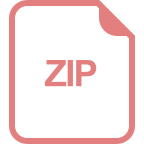
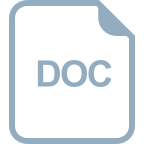
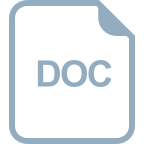
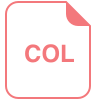
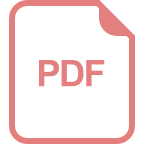
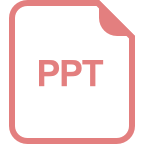
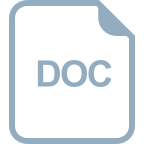
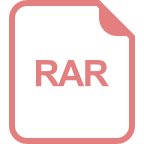
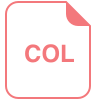
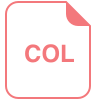
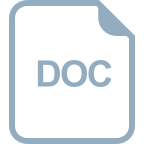
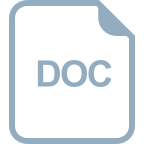
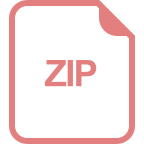
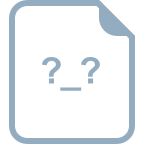
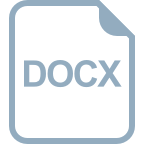
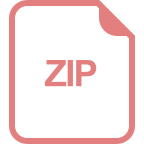
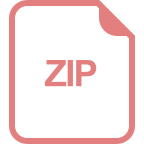