c语言写课程表的完整最简单的代码
时间: 2024-10-20 19:09:46 浏览: 21
在C语言中编写一个简单的学生课程表程序,可以使用结构体表示课程和学生,然后通过数组或链表存储数据。以下是一个基本的示例,假设每个课程只有一个学生,并且课程名和学生姓名都是字符串:
```c
#include <stdio.h>
#include <string.h>
// 定义一个结构体来存储课程信息
typedef struct {
char course[50]; // 课程名
} Course;
// 定义一个结构体来存储学生信息
typedef struct {
char student_name[50]; // 学生姓名
Course courses[10]; // 每位学生最多选修10门课程
} Student;
// 添加一门新课程到学生的课程表
void addCourse(Student* student, const char* course) {
if (student->courses_count < sizeof(student->courses)) {
strcpy(student->courses[student->courses_count].course, course);
student->courses_count++;
} else {
printf("学生已经选满课程!\n");
}
}
int main() {
// 创建一个学生并添加课程
Student student;
student.student_name = "张三";
student.courses_count = 0;
addCourse(&student, "数学");
addCourse(&student, "英语");
// 打印课程表
printf("学生 %s 的课程表:\n", student.student_name);
for (int i = 0; i < student.courses_count; i++) {
printf("%d. %s\n", i+1, student.courses[i].course);
}
return 0;
}
```
阅读全文
相关推荐



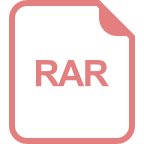
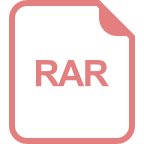



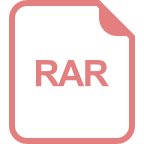

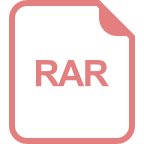
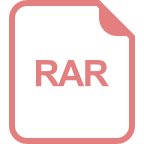

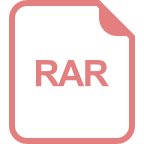




