WinForm中使用SendInput实现屏幕键盘
时间: 2024-10-21 08:16:07 浏览: 29
在Windows Form应用中,SendInput函数通常用于模拟键盘输入,这对于创建屏幕键盘或者处理需要特殊键入的情况非常有用。SendInput函数属于Windows API的一部分,可以发送模拟硬件设备生成的消息到应用程序。
以下是一个简单的步骤说明如何使用SendInput在WinForms中实现屏幕键盘:
1. **包含头文件**:
首先,在项目中引用`System.Windows.Forms`和`System.Runtime.InteropServices`命名空间,以便使用相关的类和函数。
```csharp
using System;
using System.Windows.Forms;
using System.Runtime.InteropServices;
```
2. **声明所需结构**:
SendInput操作需要使用`INPUT`结构体,它包含了键击、鼠标点击等信息。你需要声明这个结构并定义虚拟按键映射。
```csharp
[StructLayout(LayoutKind.Sequential)]
public struct INPUT
{
public InputType Type;
public int wParam;
public IntPtr lParam;
}
enum InputType : uint
{
KEYDOWN = 0x01,
KEYUP = 0x02,
.MOUSEEVENTF_LEFTDOWN = 0x02,
MOUSEEVENTF_LEFTUP = 0x04
}
```
3. **编写SendInput函数**:
实现SendInput函数来发送指定的键入事件。
```csharp
[DllImport("user32.dll", SetLastError = true)]
private static extern bool SendInput(int nInputs, INPUT[] pInputs, int cbSize);
```
4. **屏幕键盘的实现**:
创建一个控件数组来表示你的键盘布局,然后根据用户选择在数组中设置键值,并通过SendInput发送相应的键入请求。
```csharp
private void OnKeyPress(object sender, KeyPressEventArgs e)
{
// 你的键盘布局数组
char[,] virtualKeys = new char[,]
{
{'A', 'B', 'C'}, {'D', 'E', 'F'}, {'G', 'H', 'I'}
};
// 根据用户的按下事件找到对应的键位索引
int keyIndex = Array.IndexOf(virtualKeys, new char[]{e.KeyChar});
if (keyIndex != -1)
{
// 定义键入事件的INPUT结构
INPUT input = new INPUT
{
Type = InputType.KEYDOWN,
wParam = (uint)Keys.VK_CONTROL | (uint)virtualKeys.GetLength(0), // 控制键加列数作为高位键码
lParam = Marshal.StringToHGlobalUni(virtualKeys[keyIndex].ToString())
};
// 发送键入事件
if (!SendInput(1, ref input, Marshal.SizeOf(input)))
{
Console.WriteLine("SendInput failed");
}
}
}
```
记得关闭全局的SendInput操作,例如在窗体关闭时释放资源。
阅读全文
相关推荐
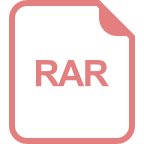
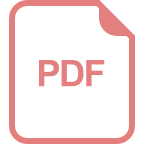
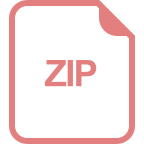
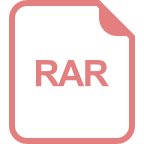
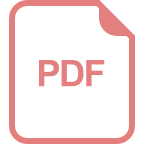
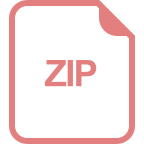
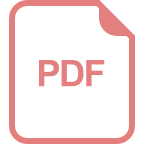
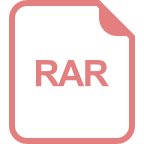
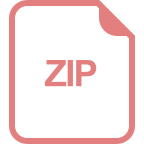
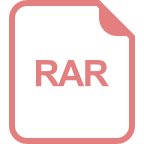
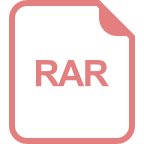
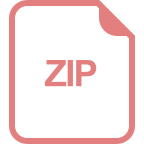
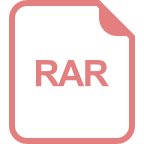
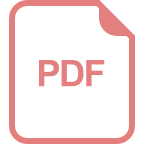