Vue写一个方法点击按钮除法是否确认弹窗
时间: 2023-03-28 16:03:28 浏览: 63
可以通过以下代码实现:
```
<template>
<div>
<button @click="handleClick">除法</button>
<div v-if="showConfirm">
<p>确认除法操作吗?</p>
<button @click="confirm">确认</button>
<button @click="cancel">取消</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
showConfirm: false
}
},
methods: {
handleClick() {
this.showConfirm = true
},
confirm() {
// 进行除法操作
this.showConfirm = false
},
cancel() {
this.showConfirm = false
}
}
}
</script>
```
当点击按钮时,会显示一个确认弹窗,询问用户是否确认进行除法操作。如果用户点击确认,则进行除法操作;如果用户点击取消,则关闭弹窗,不进行除法操作。
相关问题
帮我使用vue实现点击一个按钮可以出现一个弹窗
好的,以下是一个简单的实现点击按钮弹出弹窗的示例:
```
<template>
<div>
<button @click="showModal = true">点击打开弹窗</button>
<div class="modal" v-if="showModal">
<div class="modal-mask"></div>
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h4 class="modal-title">弹窗标题</h4>
<button type="button" class="close" @click="closeModal">
<span>×</span>
</button>
</div>
<div class="modal-body">弹窗内容</div>
<div class="modal-footer">
<button type="button" class="btn btn-secondary" @click="closeModal">关闭</button>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
showModal: false
};
},
methods: {
closeModal() {
this.showModal = false;
}
}
};
</script>
<style>
.modal {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
display: flex;
align-items: center;
justify-content: center;
z-index: 9999;
}
.modal-mask {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5);
}
.modal-dialog {
position: relative;
width: 500px;
max-width: 100%;
margin: 0 auto;
}
.modal-content {
position: relative;
background-color: #fff;
border-radius: 4px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.3);
}
.modal-header {
padding: 10px;
border-bottom: 1px solid #eee;
display: flex;
align-items: center;
justify-content: space-between;
}
.modal-title {
margin: 0;
font-size: 18px;
}
.modal-body {
padding: 10px;
}
.modal-footer {
padding: 10px;
border-top: 1px solid #eee;
display: flex;
justify-content: flex-end;
}
.btn {
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: 400;
line-height: 1.42857143;
text-align: center;
white-space: nowrap;
vertical-align: middle;
cursor: pointer;
border: 1px solid transparent;
border-radius: 4px;
user-select: none;
}
.btn-secondary {
color: #fff;
background-color: #333;
border-color: #333;
}
.btn-secondary:hover {
color: #fff;
background-color: #292929;
border-color: #292929;
}
</style>
```
使用方法:
1. 在需要使用弹窗的组件中引入该组件:
```
<template>
<div>
<button @click="showModal = true">点击打开弹窗</button>
<Modal v-if="showModal" @close="closeModal">
<div slot="header">弹窗标题</div>
<div slot="body">弹窗内容</div>
<div slot="footer">
<button class="btn btn-secondary" @click="closeModal">关闭</button>
</div>
</Modal>
</div>
</template>
<script>
import Modal from './Modal.vue';
export default {
components: {
Modal
},
data() {
return {
showModal: false
};
},
methods: {
closeModal() {
this.showModal = false;
}
}
};
</script>
```
2. 在需要使用弹窗的组件中定义一个布尔类型的变量showModal,用于控制弹窗的显示和隐藏。
3. 在需要使用弹窗的组件中定义一个方法closeModal,用于关闭弹窗并将showModal变量设置为false。
4. 在需要使用弹窗的组件中使用Modal组件,并使用slot分发header、body和footer三个插槽,用于自定义弹窗的标题、内容和底部按钮。
vue只用element ui怎么在一个表格中点击按钮弹出弹窗
你可以使用 Element UI 的 Dialog 对话框组件来实现在表格中点击按钮弹出弹窗的功能。具体实现步骤如下:
1. 在表格中添加一个操作列,该列包含一个按钮,用于触发弹窗。
2. 在按钮中绑定一个点击事件,该事件会调用一个方法。
3. 在该方法中,使用 Element UI 的 Dialog 组件来创建一个弹窗,并设置弹窗的内容。
4. 在弹窗中添加需要的表单元素或其他组件,并进行相关的操作。
下面是一个简单的代码示例,以帮助你更好地理解如何实现这个功能:
```
<template>
<el-table :data="tableData">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄"></el-table-column>
<<el-table-column label="操作">
<template slot-scope="scope">
<el-button type="primary" @click="handleClick(scope.row)">编辑</el-button>
</template>
</el-table-column>
</el-table>
<el-dialog :visible.sync="dialogVisible">
<el-form>
<el-form-item label="姓名">
<el-input v-model="editRow.name"></el-input>
</el-form-item>
<el-form-item label="年龄">
<el-input v-model="editRow.age"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取消</el-button>
<el-button type="primary" @click="saveEdit">保存</el-button>
</div>
</el-dialog>
</template>
<script>
export default {
data() {
return {
tableData: [
{ name: '张三', age: 18 },
{ name: '李四', age: 20 }
],
dialogVisible: false,
editRow: {}
}
},
methods: {
handleClick(row) {
this.editRow = Object.assign({}, row)
this.dialogVisible = true
},
saveEdit() {
// 在这里进行保存操作
this.dialogVisible = false
}
}
}
</script>
```
在这个示例中,我们在表格中添加了一个“操作”列,该列包含一个“编辑”按钮。当用户点击该按钮时,会触发 `handleClick` 方法。该方法会将当前行的数据复制到 `editRow` 对象中,并将 `dialogVisible` 属性设置为 `true`,以显示一个弹窗。
弹窗中包含一个表单,用户可以在其中编辑数据。当用户点击“保存”按钮时,会触发 `saveEdit` 方法,该方法可以在这里进行保存操作。最后,我们将 `dialogVisible` 属性设置为 `false`,以关闭弹窗。
需要注意的是,在这个示例中,我们使用了 `Object.assign` 方法来创建一个新的对象,以确保我们不会直接修改原始数据。这是一个非常重要的步骤,因为直接修改原始数据可能会导致一些意外的结果。
相关推荐
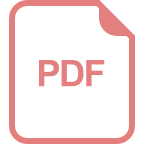
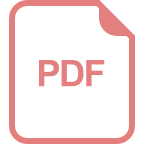












