通过肤色检测、轮廓检测、级联器多种方法组合来进行摄像头手部区域的分割代码,并限制输出图片800X600
时间: 2024-01-24 10:04:12 浏览: 28
好的,以下是基于Python和OpenCV库实现的摄像头手部区域分割的示例代码,并限制输出图片大小为800x600:
```python
import cv2
# 加载级联器
hand_cascade = cv2.CascadeClassifier('hand.xml')
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取摄像头帧
ret, frame = cap.read()
# 进行肤色检测(这里只是一个示例,实际中可能需要调整阈值等参数)
hsv = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
lower_skin = np.array([0, 20, 70], dtype=np.uint8)
upper_skin = np.array([20, 255, 255], dtype=np.uint8)
mask = cv2.inRange(hsv, lower_skin, upper_skin)
# 进行轮廓检测(这里只是一个示例,实际中可能需要调整阈值等参数)
contours, _ = cv2.findContours(mask, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 选取最大轮廓
max_contour = max(contours, key=cv2.contourArea)
# 构建矩形框并限制输出图片大小
x, y, w, h = cv2.boundingRect(max_contour)
if w > 800 or h > 600:
scale = min(800/w, 600/h)
w = int(w * scale)
h = int(h * scale)
x = int(x + (w - w*scale)/2)
y = int(y + (h - h*scale)/2)
img_crop = frame[y:y+h, x:x+w]
img_crop = cv2.resize(img_crop, (800, 600))
# 使用级联器检测手部区域(这里只是一个示例,实际中可能需要调整级联器参数)
gray = cv2.cvtColor(img_crop, cv2.COLOR_BGR2GRAY)
hands = hand_cascade.detectMultiScale(gray, 1.1, 4)
# 在原始图像上绘制矩形框
for (hx, hy, hw, hh) in hands:
cv2.rectangle(frame, (x+hx, y+hy), (x+hx+hw, y+hy+hh), (0, 255, 0), 2)
# 显示结果
cv2.imshow('Original Image', frame)
cv2.imshow('Cropped Image', img_crop)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
需要注意的是,肤色检测和轮廓检测的阈值等参数需要根据具体情况进行调整,才能得到最佳的结果。同时,级联器参数的调整也是非常重要的。
相关推荐
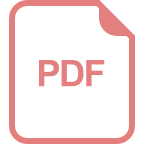
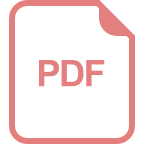
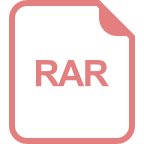














