jdk1.8中 springboot 整合minio 实现多级目录下文件的下载
时间: 2024-06-08 22:06:11 浏览: 303
首先,在Spring Boot项目中添加MinIO客户端依赖:
```xml
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>7.1.0</version>
</dependency>
```
然后,创建一个MinioClient的Bean:
```java
@Configuration
public class MinioConfig {
@Value("${minio.url}")
private String url;
@Value("${minio.accessKey}")
private String accessKey;
@Value("${minio.secretKey}")
private String secretKey;
@Value("${minio.bucketName}")
private String bucketName;
@Bean
public MinioClient minioClient() throws InvalidEndpointException, InvalidPortException {
return MinioClient.builder()
.endpoint(url)
.credentials(accessKey, secretKey)
.build();
}
@Bean
public String bucketName() {
return bucketName;
}
}
```
其中,`accessKey`和`secretKey`是在MinIO服务器上创建的AccessKey和SecretKey,`bucketName`是要操作的存储桶名称。
接下来,实现多级目录下文件的下载。假设我们要下载的文件路径为`/parent/child/test.txt`,那么可以使用以下代码:
```java
@Autowired
private MinioClient minioClient;
@Autowired
private String bucketName;
@GetMapping("/download")
public void downloadFile(HttpServletResponse response) throws Exception {
String objectName = "parent/child/test.txt";
InputStream inputStream = minioClient.getObject(bucketName, objectName);
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=\"" + objectName + "\"");
IOUtils.copy(inputStream, response.getOutputStream());
response.flushBuffer();
}
```
其中,`minioClient.getObject(bucketName, objectName)`用于获取文件的输入流,`IOUtils.copy(inputStream, response.getOutputStream())`用于将输入流复制到响应的输出流中,从而实现文件的下载。
需要注意的是,如果要下载的文件路径中包含中文字符,需要进行URL编码,例如:
```java
String encodedObjectName = URLEncoder.encode("parent/child/测试.txt", "UTF-8");
InputStream inputStream = minioClient.getObject(bucketName, encodedObjectName);
```
阅读全文
相关推荐
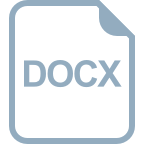
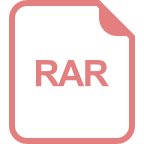
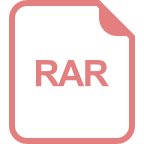
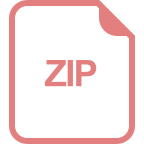
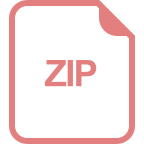
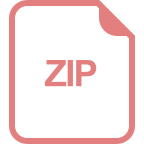
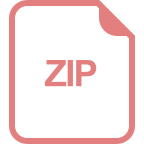
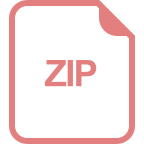
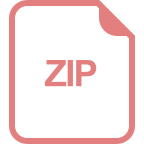
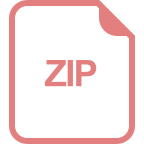
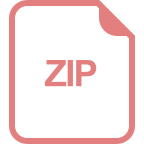
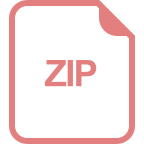
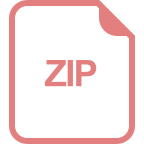