在Linux下用C语言完成以下任务,首先随机生成10个100以内的整数,并将这些数存放在名为data.txt的文件中。之后创建三个进程和两个管道。进程一的任务是读出data.txt中的数据并将其写入管道一;进程二的任务是从管道一中取出数据,并对它们进行求和,将新的结果写入管道二;进程三的任务是从管道二中取出数据并将其保存在新的名为result.txt的文件中,请给出具体的C语言代码。
时间: 2024-03-27 19:37:18 浏览: 58
下面是实现以上任务的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
#define MAX_NUM 10
#define MAX_VALUE 100
int main() {
int i;
int nums[MAX_NUM];
FILE *fp;
pid_t pid1, pid2, pid3;
int fd1[2], fd2[2], status;
int sum = 0;
// 随机生成10个100以内的整数
srand(time(NULL));
for (i = 0; i < MAX_NUM; i++) {
nums[i] = rand() % MAX_VALUE;
}
// 将这些数存放在名为data.txt的文件中
fp = fopen("data.txt", "w");
for (i = 0; i < MAX_NUM; i++) {
fprintf(fp, "%d\n", nums[i]);
}
fclose(fp);
// 创建管道一
if (pipe(fd1) < 0) {
perror("pipe error");
exit(1);
}
// 创建管道二
if (pipe(fd2) < 0) {
perror("pipe error");
exit(1);
}
// 创建进程一
pid1 = fork();
if (pid1 < 0) {
perror("fork error");
exit(1);
} else if (pid1 == 0) { // 子进程一
close(fd1[0]); // 关闭管道一的读端
fp = fopen("data.txt", "r");
char buf[10];
while (fgets(buf, 10, fp) != NULL) {
write(fd1[1], buf, 10); // 将数据写入管道一
}
fclose(fp);
close(fd1[1]); // 关闭管道一的写端
exit(0);
}
// 创建进程二
pid2 = fork();
if (pid2 < 0) {
perror("fork error");
exit(1);
} else if (pid2 == 0) { // 子进程二
close(fd1[1]); // 关闭管道一的写端
close(fd2[0]); // 关闭管道二的读端
char buf[10];
while (read(fd1[0], buf, 10) > 0) {
sum += atoi(buf); // 将数据进行求和
}
write(fd2[1], &sum, sizeof(sum)); // 将新的结果写入管道二
close(fd1[0]); // 关闭管道一的读端
close(fd2[1]); // 关闭管道二的写端
exit(0);
}
// 创建进程三
pid3 = fork();
if (pid3 < 0) {
perror("fork error");
exit(1);
} else if (pid3 == 0) { // 子进程三
close(fd2[1]); // 关闭管道二的写端
fp = fopen("result.txt", "w");
int result;
read(fd2[0], &result, sizeof(result)); // 从管道二中取出数据
fprintf(fp, "sum=%d\n", result); // 将数据保存在新的名为result.txt的文件中
fclose(fp);
close(fd2[0]); // 关闭管道二的读端
exit(0);
}
// 等待子进程的退出
waitpid(pid1, &status, 0);
waitpid(pid2, &status, 0);
waitpid(pid3, &status, 0);
return 0;
}
```
阅读全文
相关推荐
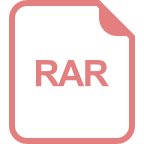
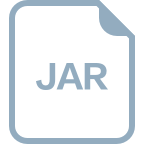
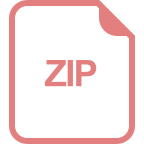
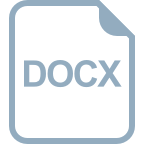
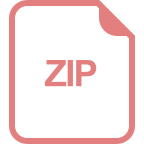
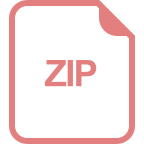
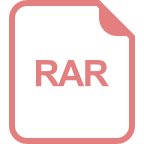
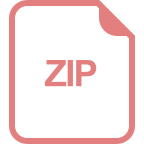
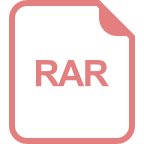