相机sdk开发 opencv
时间: 2023-08-25 12:07:36 浏览: 131
要在相机应用中使用 OpenCV,您需要使用相机 SDK 将图像从相机读取到您的应用程序中,然后使用 OpenCV 对这些图像进行处理。以下是一些常用的相机 SDK 和 OpenCV 的集成步骤:
1. 使用相机 SDK 初始化相机,设置相机参数并打开相机。
2. 编写回调函数来处理从相机读取的图像数据。
3. 在回调函数中将相机图像转换为 OpenCV 图像格式。
4. 使用 OpenCV 对相机图像进行处理,并将处理后的图像传回相机应用程序进行显示或保存。
以下是一个示例代码,它使用 Point Grey 的相机 SDK 和 OpenCV 库来捕获和处理相机图像:
```cpp
#include "FlyCapture2.h"
#include "opencv2/opencv.hpp"
using namespace FlyCapture2;
using namespace cv;
int main()
{
// Initialize the camera
Camera camera;
CameraInfo camInfo;
Error error = camera.Connect(0);
if (error != PGRERROR_OK) {
std::cerr << "Failed to connect to camera" << std::endl;
return -1;
}
error = camera.GetCameraInfo(&camInfo);
if (error != PGRERROR_OK) {
std::cerr << "Failed to get camera info from camera" << std::endl;
return -1;
}
std::cout << "Connected to camera: " << camInfo.modelName << std::endl;
// Set camera parameters
VideoMode videoMode = VIDEOMODE_640x480Y8;
FrameRate frameRate = FRAMERATE_30;
error = camera.SetVideoModeAndFrameRate(videoMode, frameRate);
if (error != PGRERROR_OK) {
std::cerr << "Failed to set video mode and frame rate" << std::endl;
return -1;
}
// Start capturing images
error = camera.StartCapture();
if (error != PGRERROR_OK) {
std::cerr << "Failed to start capture" << std::endl;
return -1;
}
// Loop to capture and process images
while (true) {
// Retrieve an image from the camera
Image rawImage;
error = camera.RetrieveBuffer(&rawImage);
if (error != PGRERROR_OK) {
std::cerr << "Failed to retrieve buffer from camera" << std::endl;
continue;
}
// Convert the raw image to OpenCV format
Mat image(rawImage.GetRows(), rawImage.GetCols(), CV_8UC1, rawImage.GetData(), rawImage.GetStride());
cvtColor(image, image, COLOR_BayerGR2BGR);
// Process the image with OpenCV
cv::GaussianBlur(image, image, Size(5, 5), 0);
// Display the processed image
cv::imshow("Processed Image", image);
if (waitKey(1) == 27) break; // Exit on ESC key
}
// Stop capturing images
error = camera.StopCapture();
if (error != PGRERROR_OK) {
std::cerr << "Failed to stop capture" << std::endl;
return -1;
}
// Disconnect the camera
camera.Disconnect();
return 0;
}
```
在这个示例中,我们使用 Point Grey 的相机 SDK 和 OpenCV 库来捕获相机图像并对其进行高斯模糊。在每次循环中,我们将相机图像转换为 OpenCV 格式,然后使用 `cv::GaussianBlur` 函数对其进行处理。最后,我们将处理后的图像显示在窗口中,并等待 ESC 键退出循环。
阅读全文
相关推荐
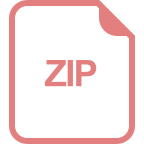
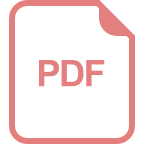
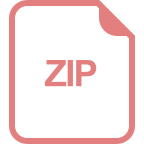
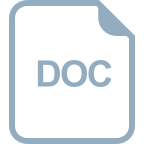

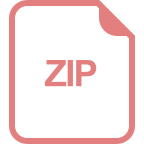
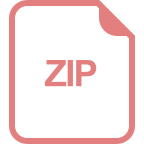
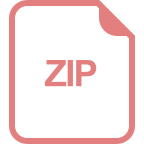
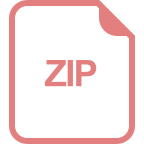
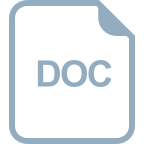
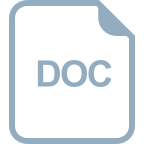
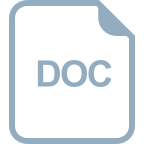


