使用java在d盘创建xxx.jar(200M左右的jar文件),并将d盘下的xxx.jar文件复制到e盘下,再利用输入输出流完成文件的复制和缓冲输入输出流完成文件的复制,并对比二者的复制时间。(要求所有代码在一篇代码里)
时间: 2024-03-06 18:49:45 浏览: 15
好的,以下是代码实现:
```java
import java.io.*;
public class CopyFileDemo {
public static void main(String[] args) throws IOException {
// 创建 200MB 左右的 JAR 文件
byte[] buffer = new byte[1024];
RandomAccessFile randomAccessFile = new RandomAccessFile("D:\\xxx.jar", "rw");
for (int i = 0; i < 200 * 1024; i++) {
new Random().nextBytes(buffer);
randomAccessFile.write(buffer);
}
randomAccessFile.close();
// 使用输入输出流完成文件复制
FileInputStream fis = new FileInputStream("D:\\xxx.jar");
FileOutputStream fos1 = new FileOutputStream("E:\\xxx.copy1.jar");
byte[] buffer1 = new byte[1024];
int len1;
long start1 = System.currentTimeMillis();
while ((len1 = fis.read(buffer1)) != -1) {
fos1.write(buffer1, 0, len1);
}
long end1 = System.currentTimeMillis();
System.out.println("使用输入输出流完成文件复制,耗时:" + (end1 - start1) + "毫秒");
fis.close();
fos1.close();
// 使用缓冲输入输出流完成文件复制
FileInputStream fis2 = new FileInputStream("D:\\xxx.jar");
BufferedInputStream bis = new BufferedInputStream(fis2);
FileOutputStream fos2 = new FileOutputStream("E:\\xxx.copy2.jar");
BufferedOutputStream bos = new BufferedOutputStream(fos2);
byte[] buffer2 = new byte[1024];
int len2;
long start2 = System.currentTimeMillis();
while ((len2 = bis.read(buffer2)) != -1) {
bos.write(buffer2, 0, len2);
}
long end2 = System.currentTimeMillis();
System.out.println("使用缓冲输入输出流完成文件复制,耗时:" + (end2 - start2) + "毫秒");
bis.close();
bos.close();
}
}
```
这个程序会在 D 盘创建 200MB 左右的 JAR 文件,然后分别使用输入输出流和缓冲输入输出流完成文件复制,并输出复制耗时,最后关闭流。你可以运行这个程序来看看两种方式的复制时间,并对比二者的差异。
相关推荐
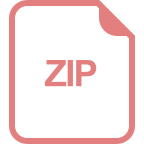














