编写手机类(MobilePhone),含有type(型号,String类型)、price(价格,int类型)属性,在链表中添加n个手机对象(从键盘输入),并实现以下操作:1)按手机价格从低到高输出手机信息。2)实现根据型号查找手机信息。3)查找某个价格区间内的手机(如2000~3000
时间: 2023-06-26 11:06:57 浏览: 317
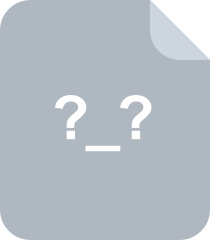
php 手机类的实现
下面是一个可能的实现:
```java
import java.util.Scanner;
class MobilePhone {
private String type;
private int price;
public MobilePhone(String type, int price) {
this.type = type;
this.price = price;
}
public String getType() {
return type;
}
public int getPrice() {
return price;
}
public String toString() {
return type + " " + price;
}
}
class Node {
public MobilePhone data;
public Node next;
public Node(MobilePhone data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
private Node head;
public void add(MobilePhone data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void print() {
Node current = head;
while (current != null) {
System.out.println(current.data);
current = current.next;
}
}
public MobilePhone findByType(String type) {
Node current = head;
while (current != null) {
if (current.data.getType().equals(type)) {
return current.data;
}
current = current.next;
}
return null;
}
public void findByPrice(int minPrice, int maxPrice) {
Node current = head;
while (current != null) {
if (current.data.getPrice() >= minPrice && current.data.getPrice() <= maxPrice) {
System.out.println(current.data);
}
current = current.next;
}
}
public void sortByPrice() {
Node current = head;
while (current != null) {
Node minNode = current;
Node innerCurrent = current.next;
while (innerCurrent != null) {
if (innerCurrent.data.getPrice() < minNode.data.getPrice()) {
minNode = innerCurrent;
}
innerCurrent = innerCurrent.next;
}
if (current != minNode) {
MobilePhone tmp = current.data;
current.data = minNode.data;
minNode.data = tmp;
}
current = current.next;
}
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入手机个数:");
int n = scanner.nextInt();
LinkedList list = new LinkedList();
for (int i = 0; i < n; i++) {
System.out.print("请输入第" + (i+1) + "个手机型号和价格:");
String type = scanner.next();
int price = scanner.nextInt();
MobilePhone phone = new MobilePhone(type, price);
list.add(phone);
}
System.out.println("按价格从低到高排序后的手机列表:");
list.sortByPrice();
list.print();
System.out.print("请输入要查找的手机型号:");
String type = scanner.next();
MobilePhone phone = list.findByType(type);
if (phone != null) {
System.out.println("找到了该型号的手机:" + phone);
} else {
System.out.println("没有找到该型号的手机。");
}
System.out.print("请输入要查找的价格区间(最低价格和最高价格,用空格隔开):");
int minPrice = scanner.nextInt();
int maxPrice = scanner.nextInt();
System.out.println("价格在" + minPrice + "元和" + maxPrice + "元之间的手机有:");
list.findByPrice(minPrice, maxPrice);
}
}
```
这个程序中,MobilePhone 表示手机类,LinkedList 表示链表类,Node 表示链表中的节点。在 main 函数中,首先从键盘输入 n 个手机对象,并添加到链表中。然后按照价格从低到高排序,并输出所有手机的信息。接下来,从键盘输入要查找的手机型号和价格区间,并使用链表中的方法进行查找。注意,为了方便起见,这里只实现了按价格从低到高排序,而没有实现按价格从高到低排序。
阅读全文
相关推荐
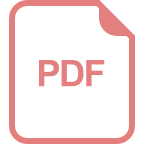




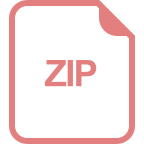
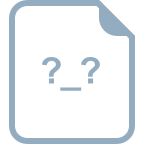
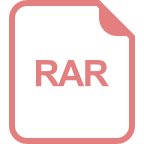
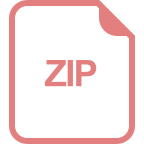



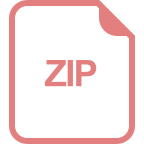
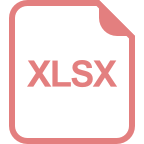