某小区物业为了更好的服务住户,为空巢老人和单亲家庭发放社区购物优惠证。空巢老人优惠证和单亲家庭可以享受购物打折优惠,具体优惠力度视家庭情况而定,空巢老人优惠证在8折的基础上,每多一个老人折扣减1到1.5之间不定(最多两个老人),单亲家庭优惠证在9折的基础上,每多一个孩子,折扣减0.5-1之间不定(最多5个孩子)。持证到物业处,输入家庭单人享受的减少折扣,可以查询优惠证的折扣力度。请依据题目完成下列题目: (1)设计优惠证抽象类Discount,包含家庭类型和享受优惠人数两个封装属性,为属性添加setter和getter方法;定义抽象方法返回优惠证可每次消费享受的折扣费用 public abstract double getDiscount(int peopleCount);其中peopleCount为单人享受的折扣减少折扣。(20分) (2)创建子类EmptyNestDiscount和SingleParentDiscount继承Discount类并实现getDiscount方法,依据优惠证类型和单人享受的减少折扣返回此优惠证的最终享受折扣(最终享受折扣=优惠证折扣基础 - 单人享受的减少折扣 * 享受优惠人数)(例如:空巢老人优惠证的单人享受减少折扣是1.2,家庭人数是2,那最终折扣为8-1.2*2=5.6折)。(20分) (3)在测试主类TestDiscount中以你的名字和张三创建两个优惠证,分别为空巢优惠证和单亲优惠证,分别设置家庭类型和享受优惠人数。(10分) (4)在测试主类TestDiscount创建static类型getInfo方法:public static void getInfo(Discount dc,double peopleDiscount)显示优惠证类别和优惠证的折扣力度。(10分)
时间: 2024-02-03 07:12:02 浏览: 100
(1) 抽象类Discount的代码如下:
```java
public abstract class Discount {
private String familyType; // 家庭类型
private int peopleCount; // 享受优惠人数
public String getFamilyType() {
return familyType;
}
public void setFamilyType(String familyType) {
this.familyType = familyType;
}
public int getPeopleCount() {
return peopleCount;
}
public void setPeopleCount(int peopleCount) {
this.peopleCount = peopleCount;
}
public abstract double getDiscount(int peopleCount);
}
```
(2) EmptyNestDiscount和SingleParentDiscount的代码如下:
```java
public class EmptyNestDiscount extends Discount {
@Override
public double getDiscount(int peopleCount) {
double baseDiscount = 8.0;
if (getPeopleCount() == 1) {
return baseDiscount - peopleCount;
} else if (getPeopleCount() == 2) {
return baseDiscount - peopleCount * 1.5;
} else {
return baseDiscount;
}
}
}
public class SingleParentDiscount extends Discount {
@Override
public double getDiscount(int peopleCount) {
double baseDiscount = 9.0;
if (getPeopleCount() == 1) {
return baseDiscount - peopleCount;
} else if (getPeopleCount() == 2) {
return baseDiscount - peopleCount * 1.0;
} else if (getPeopleCount() == 3) {
return baseDiscount - peopleCount * 0.5;
} else if (getPeopleCount() == 4) {
return baseDiscount - peopleCount * 0.75;
} else if (getPeopleCount() == 5) {
return baseDiscount - peopleCount * 1.0;
} else {
return baseDiscount;
}
}
}
```
(3) TestDiscount的代码如下:
```java
public class TestDiscount {
public static void main(String[] args) {
EmptyNestDiscount emptyNestDiscount = new EmptyNestDiscount();
emptyNestDiscount.setFamilyType("empty nest");
emptyNestDiscount.setPeopleCount(2);
SingleParentDiscount singleParentDiscount = new SingleParentDiscount();
singleParentDiscount.setFamilyType("single parent");
singleParentDiscount.setPeopleCount(4);
getInfo(emptyNestDiscount, 1.2);
getInfo(singleParentDiscount, 0.5);
}
public static void getInfo(Discount dc, double peopleDiscount) {
String discountType = dc.getFamilyType();
double finalDiscount = dc.getDiscount((int) peopleDiscount);
System.out.println("Discount type: " + discountType);
System.out.println("Final discount: " + finalDiscount);
}
}
```
阅读全文
相关推荐
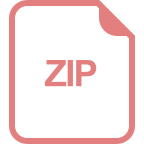
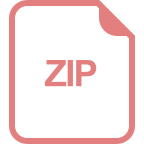
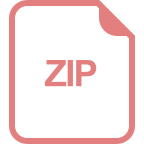

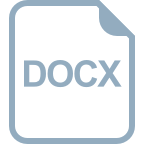
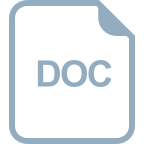
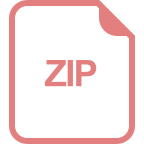
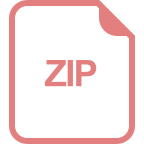
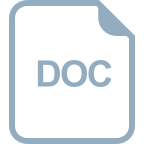
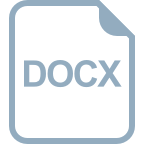
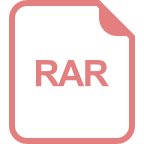
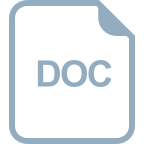
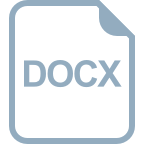
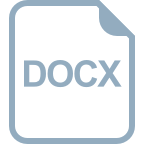
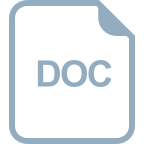
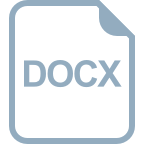
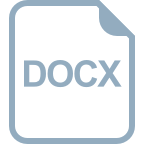
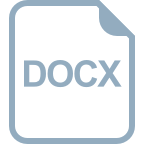