c# 浮点数保存到文件
时间: 2024-10-17 19:12:42 浏览: 9
在 C# 中,浮点数(如 double 或 float 类型)可以安全地保存到文件中,通常我们会序列化这些数据以便存储。你可以使用 `System.IO` 包中的 `BinaryFormatter` 或者 `StreamWriter` 来实现。以下是简单的示例:
1. 使用 `BinaryFormatter` 示例:
```csharp
using System;
using System.IO;
public class Program
{
public static void Main(string[] args)
{
// 浮点数变量
double value = 3.14159;
// 创建一个内存流
using (MemoryStream ms = new MemoryStream())
{
// 创建 BinaryFormatter 实例
BinaryFormatter formatter = new BinaryFormatter();
// 序列化浮点数
formatter.Serialize(ms, value);
// 将内存流的数据写入文件
File.WriteAllBytes("float_value.bin", ms.ToArray());
}
Console.WriteLine("Value saved to file.");
}
}
```
2. 使用 `StreamWriter` 示例:
```csharp
using System;
using System.IO;
public class Program
{
public static void Main(string[] args)
{
// 浮点数变量
double value = 3.14159;
// 写入文件
using (StreamWriter writer = new StreamWriter("float_value.txt"))
{
// 转换为字符串并写入
writer.WriteLine(value);
}
Console.WriteLine("Value saved to file as text.");
}
}
```
在读取时,你可以反向操作,使用相同的策略从文件中恢复数据。
阅读全文
相关推荐
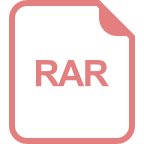
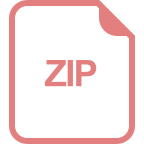
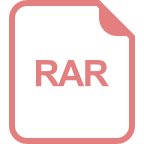















