tkinter的listbox().size
时间: 2023-11-11 20:05:47 浏览: 32
在 tkinter 中,Listbox 控件的 size() 方法用于获取 Listbox 中当前项目的数量。该方法返回一个整数,表示 Listbox 中项目的数量。以下是使用 Listbox 的 size() 方法的示例:
```
import tkinter as tk
root = tk.Tk()
# 创建 Listbox 控件并添加一些项目
listbox = tk.Listbox(root)
for i in range(10):
listbox.insert(tk.END, f"Item {i+1}")
listbox.pack()
# 获取 Listbox 中项目的数量
num_items = listbox.size()
print(f"Number of items in Listbox: {num_items}")
root.mainloop()
```
在这个示例中,我们创建了一个 Listbox 控件并添加了 10 个项目。然后,我们使用 size() 方法获取 Listbox 中项目的数量,并打印输出该数量。输出应该类似于以下内容:
```
Number of items in Listbox: 10
```
相关问题
tkinter Listbox
tkinter Listbox是一个列表框控件,可以在其中插入文本项,并可以设置为单选或多选。可以使用insert方法向Listbox中插入item,使用size方法得到当前Listbox中的item个数。此外,还可以通过listvariable参数将Listbox与变量进行绑定。绑定后,可以使用get方法获取与Listbox绑定的变量的值。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Python tkinter编程之Listbox篇](https://blog.csdn.net/qiukapi/article/details/104067586)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
tkinter listbox如何设置字体大小
你可以使用 `Listbox` 的 `font` 属性来设置字体大小。你需要使用 `tkFont.Font()` 方法创建一个新的字体对象,并将其作为参数传递给 `font` 属性。
下面的代码演示了如何设置 `Listbox` 中的文本字体大小:
```python
import tkinter as tk
import tkinter.font as tkFont
root = tk.Tk()
# 创建字体对象
my_font = tkFont.Font(family='Helvetica', size=16)
listbox = tk.Listbox(root, font=my_font)
listbox.pack()
# 添加一些文本项
for i in range(10):
listbox.insert(tk.END, f"Item {i}")
root.mainloop()
```
在这个例子中,我们创建了一个名为 `my_font` 的字体对象,它使用 Helvetica 字体,大小为 16。然后,我们将这个字体对象传递给 `Listbox` 的 `font` 属性。
你可以根据自己的需求调整字体的大小和样式。
相关推荐
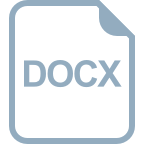





import re,tkinter,requests,threading,tqdm as tt root = tkinter.Tk() root.title('在线视频解析') root.geometry('500x590+550+350') headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:109.0) Gecko/20100101 Firefox/115.0'} ac = tkinter.Listbox(root, width=50, height=20, font=('黑体', 12)) ac.grid(row=2, columnspan=10, sticky="n" + "s" + "w" + "e") def sousuo(): i = b1.get() ac.delete(0, 'end') def extract_music_info(content): p = '|' content = re.sub(p, '', content, flags=re.S) pattern = re.compile('subject.*?href="(.*?)">(.*?)
', flags=re.S) return pattern.findall(content) def search_music(): url = 'https://www.hifini.com/search-' + i + '-1.htm' response = requests.get(url=url, headers=headers) return response.text def update_listbox(music_list): for music in music_list: pppp = music[1] + ":" + music[0] ac.insert('end', pppp) content = search_music() music_list = extract_music_info(content) update_listbox(music_list) def xiazzi(): def download_music(): ppp = ac.get(ac.curselection()) pp = re.search('thread.*?htm', ppp) v = pp.group() url1 = 'https://www.hifini.com/' + v response = requests.get(url=url1, headers=headers) ppp = response.text l2 = re.search('<script>.*?title:..(.*?).,.*?author:.(.*?).,.*?url:..(.*?).,', ppp, flags=re.S) p = 'https://www.hifini.com/' + l2.group(3) response = requests.get(url=p, headers=headers, stream=True) # 设置 stream=True 以启用流式下载 total_size = int(response.headers.get('Content-Length')) music_name = '{}-{}.mp3'.format(l2.group(2), l2.group(1)) progress_bar = tt.tqdm(total=total_size, unit='B', unit_scale=True) # 创建进度条 with open(music_name, 'wb') as f: for data in response.iter_content(chunk_size=1024): progress_bar.update(len(data)) # 更新进度条 f.write(data) progress_bar.close() # 关闭进度条 print(music_name) threading.Thread(target=download_music).start() a1 = tkinter.Label(root, text='音乐下载器', anchor="center", font=('黑体', 24)) a1.grid(row=0, columnspan=10, sticky="n" + "s" + "w" + "e") b1 = tkinter.Entry(root, width=35, font=('黑体', 16), ) b1.grid(row=1, column=3, padx=15) search_button = tkinter.Button(root, text='搜索', command=sousuo) search_button.grid(row=1, column=4) download_button = tkinter.Button(root, text='下载', command=xiazzi) download_button.grid(row=3, column=4) root.mainloop() 将download_button带有下载行为的按钮添加进列表,






