写一个用mmap在内存中替换字符串的函数
时间: 2024-01-22 15:20:48 浏览: 26
可以使用 mmap 函数将文件映射到进程的地址空间中,然后使用指针进行读写操作,实现字符串替换。
以下是一个使用 mmap 替换字符串的函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <fcntl.h>
#include <sys/mman.h>
#include <sys/stat.h>
void replace_string_with_mmap(char *filename, char *old_str, char *new_str) {
int fd = open(filename, O_RDWR); // 打开文件
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
struct stat st;
if (fstat(fd, &st) == -1) { // 获取文件大小
perror("fstat");
exit(EXIT_FAILURE);
}
char *map = mmap(NULL, st.st_size, PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0); // 将文件映射到内存中
if (map == MAP_FAILED) {
perror("mmap");
exit(EXIT_FAILURE);
}
char *pos = strstr(map, old_str); // 定位字符串
while (pos != NULL) { // 循环替换
memcpy(pos, new_str, strlen(new_str));
pos = strstr(pos + strlen(new_str), old_str);
}
if (munmap(map, st.st_size) == -1) { // 解除映射
perror("munmap");
exit(EXIT_FAILURE);
}
close(fd); // 关闭文件
}
```
这个函数接受三个参数:文件名、要替换的字符串和替换后的字符串。它首先打开文件,然后使用 fstat 函数获取文件大小,接着使用 mmap 将文件映射到内存中,并定位要替换的字符串。循环遍历字符串,使用 memcpy 函数进行替换。最后,使用 munmap 解除映射,关闭文件。
注意,这个函数只能用于替换较小的文件,因为将整个文件映射到内存中可能会耗费大量的内存。如果文件太大,则需要使用其他方法进行处理。
相关推荐
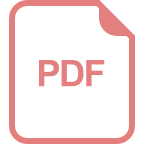














