python smtp 附件
时间: 2023-06-22 21:26:12 浏览: 96
您好,要使用 Python 发送带附件的电子邮件,可以使用 Python 内置的 smtplib 和 email 模块。以下是示例代码:
```python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.application import MIMEApplication
# 发件人邮箱地址
sender = 'your_email@example.com'
# 收件人邮箱地址
receiver = 'recipient_email@example.com'
# 邮件主题
subject = 'Email with Attachment'
# 邮件正文
body = 'This email contains an attachment.'
# 创建一个带附件的邮件实例
msg = MIMEMultipart()
msg['From'] = sender
msg['To'] = receiver
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
# 添加附件
with open('attachment.txt', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='txt')
attachment.add_header('Content-Disposition', 'attachment', filename='attachment.txt')
msg.attach(attachment)
# 发送邮件
try:
smtp_obj = smtplib.SMTP('smtp.example.com', 587)
smtp_obj.starttls()
smtp_obj.login(sender, 'password')
smtp_obj.sendmail(sender, receiver, msg.as_string())
print('Email with attachment sent successfully!')
except Exception as e:
print('Error sending email: ', e)
finally:
smtp_obj.quit()
```
在上面的代码中,您需要将 `sender` 和 `receiver` 变量替换为实际的电子邮件地址,将 `subject` 和 `body` 变量设置为您想要的主题和正文内容。您还需要将 `smtp_obj` 变量中的 SMTP 服务器地址和端口号替换为您的实际值,并将 `sender` 和 `password` 替换为您的实际发件人电子邮件地址和密码。最后,您需要将附件的文件名和路径替换为您的实际附件文件名和路径。
阅读全文
相关推荐
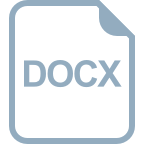
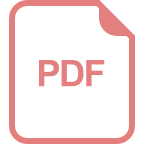
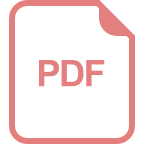
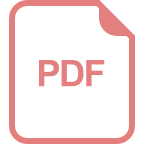
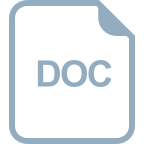
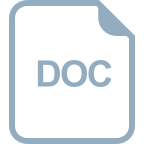
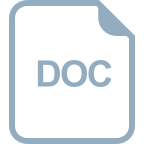
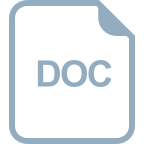
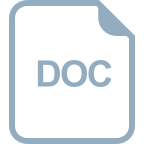
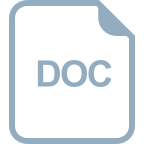





