onMounted onBeforeUnmount
时间: 2024-09-03 14:03:17 浏览: 56
Vue.js中的`onMounted`和`onBeforeUnmount`是两个生命周期钩子函数,分别用于组件的不同阶段:
1. `onMounted()`:当组件首次渲染完成并且DOM已经插入到文档中之后,会触发这个钩子。你可以在这个时候进行一些依赖DOM的操作,例如数据绑定、事件监听等。这是一个异步函数,因为它会在虚拟DOM更新并同步到实际DOM后运行。
2. `onBeforeUnmount()`:在组件即将卸载之前调用,这时组件实例仍处于活动状态,但其DOM已经被移除。你可以在这个阶段清理资源、解除事件监听等操作,以防止内存泄漏。
这两个钩子都是在Vue组件的生命周期中使用的,对于维护组件的状态和资源管理非常重要。
相关问题
<template> <div class="menu" ref="nav" :class="{ fixed_menu: isFixed }"> </div> </template><script setup lang="ts"> import { reactive, ref, onMounted, onBeforeUnmount } from "vue"; const nav = ref(null); const isFixed = ref(false); const handleScroll = () => { const { top } = nav.value.getBoundingClientRect(); console.log(top, "top"); if (top < 0) { isFixed.value = true; } else { isFixed.value = false; } }; onMounted(() => { window.addEventListener("scroll", handleScroll); });<style lang="scss" scoped> </script>.menu { width: 100%; display: flex; height: 95px; background: #f6f7fa; color: #6c7088; padding-left: 264px; border-bottom: 1px solid rgb(222, 223, 228);} .fixed_menu { position: fixed; top: 0; background: rgba(246, 247, 250, 0.7); backdrop-filter: blur(20px); } onBeforeUnmount(() => { window.removeEventListener("scroll", handleScroll); });</style> 解决页面抖动问题
页面抖动问题通常是由于滚动事件监听不当或处理不当引起的。可以尝试优化以下几个方面:
1. 减少处理滚动事件的频率,可以使用防抖或节流函数来限制触发滚动事件的频率。
2. 避免在滚动事件处理函数中进行复杂的计算或操作,尽可能简化滚动事件处理函数。
3. 避免在滚动事件处理函数中直接修改 DOM 样式,而是通过修改状态或属性来改变样式,这样可以避免重新计算布局和重新渲染导致的页面抖动问题。
在上述代码中,可以尝试将 handleScroll 函数中的 DOM 操作改为修改状态或属性,例如将 isFixed.value 直接绑定到 nav 的 class 上,避免在处理函数中频繁修改 DOM。同时,可以考虑使用 throttle 函数来限制 handleScroll 函数的触发频率。
// draggable.js import { onMounted, onBeforeUnmount } from 'vue' export default { mounted(el) { el.setAttribute('draggable', true) let startX, startY, initialLeft, initialTop, currentX, currentY const onDragStart = (e) => { e.stopPropagation() startX = e.clientX || e.touches[0].clientX startY = e.clientY || e.touches[0].clientY initialLeft = el.offsetLeft initialTop = el.offsetTop el.classList.add('dragging') } const onDrag = (e) => { e.stopPropagation() currentX = (e.clientX || e.touches[0].clientX) - startX currentY = (e.clientY || e.touches[0].clientY) - startY el.style.left = `${initialLeft + currentX}px` el.style.top = `${initialTop + currentY}px` } const onDragEnd = (e) => { e.stopPropagation() el.classList.remove('dragging') } el.addEventListener('dragstart', onDragStart) el.addEventListener('drag', onDrag) el.addEventListener('dragend', onDragEnd) el.addEventListener('touchstart', onDragStart) el.addEventListener('touchmove', onDrag) el.addEventListener('touchend', onDragEnd) onMounted(() => { el.classList.add('draggable') }) onBeforeUnmount(() => { el.removeEventListener('dragstart', onDragStart) el.removeEventListener('drag', onDrag) el.removeEventListener('dragend', onDragEnd) el.removeEventListener('touchstart', onDragStart) el.removeEventListener('touchmove', onDrag) el.removeEventListener('touchend', onDragEnd) el.classList.remove('draggable') el.classList.remove('dragging') }) } } 解决这个报错 TypeError: Cannot read properties of undefined (reading '0')
在这段代码中,并没有显示的报错信息,所以很难确定你遇到的报错是哪个。但是,根据你提供的代码以及报错信息,我们可以猜测,可能是在这行代码中出现了问题:
```
startX = e.clientX || e.touches[0].clientX
```
由于此行代码使用了逻辑或运算符,如果 `e.clientX` 为 undefined,则会尝试读取 `e.touches[0].clientX`,但是 `e.touches` 可能为空,这就会导致报错 `Cannot read properties of undefined (reading '0')`,因为你无法从一个未定义的对象中读取属性。
为了解决这个问题,你可以在使用逻辑或运算符之前,先检查 `e` 对象中的属性是否存在。例如,你可以这样修改代码:
```
startX = e.clientX || (e.touches && e.touches[0] && e.touches[0].clientX)
startY = e.clientY || (e.touches && e.touches[0] && e.touches[0].clientY)
```
这样,如果 `e.clientX` 为 undefined,就会尝试读取 `e.touches[0].clientX`,但是在读取之前,会先检查 `e.touches` 是否存在,并且检查 `e.touches[0]` 是否存在,以避免出现上述报错。
阅读全文
相关推荐
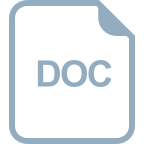
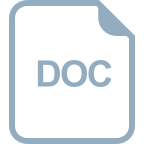
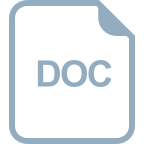




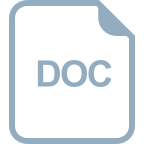
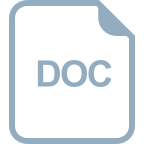
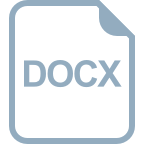
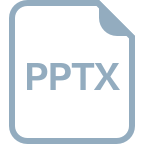
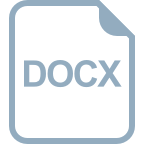
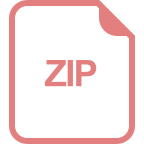
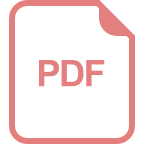
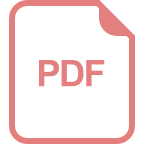
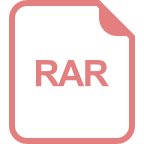
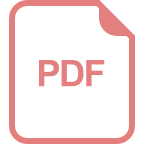