对字符串进行拷贝的函数方法
时间: 2023-12-16 16:05:33 浏览: 34
在C++中,可以使用以下几种方法来拷贝字符串:
1. 使用strcpy()函数进行拷贝:
```c++
#include <cstring>
#include <iostream>
int main() {
char source[] = "hello world";
char destination[20];
std::strcpy(destination, source);
std::cout << destination << std::endl;
return 0;
}
```
2. 使用循环遍历字符串进行拷贝:
```c++
#include <iostream>
int main() {
char source[] = "hello world";
char destination[20];
int i = 0;
while (source[i] != '\0') {
destination[i] = source[i];
i++;
}
destination[i] = '\0';
std::cout << destination << std::endl;
return 0;
}
```
3. 使用STL中的string类进行拷贝:
```c++
#include <iostream>
#include <string>
int main() {
std::string source = "hello world";
std::string destination = source;
std::cout << destination << std::endl;
return 0;
}
```
注意:使用以上三种方法进行字符串拷贝时,需要确保目标字符串有足够的空间来存储源字符串。
相关推荐
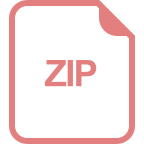














