python调用DeviceIoControl函数
时间: 2024-05-15 15:15:40 浏览: 339
在Python中调用DeviceIoControl函数需要使用ctypes库。以下是示例代码:
```
import ctypes
# 定义常量
GENERIC_READ = 0x80000000
GENERIC_WRITE = 0x40000000
FILE_SHARE_READ = 0x00000001
FILE_SHARE_WRITE = 0x00000002
OPEN_EXISTING = 3
IOCTL_DISK_GET_DRIVE_GEOMETRY = 0x70000
# 定义结构体
class DISK_GEOMETRY(ctypes.Structure):
_fields_ = [("Cylinders", ctypes.c_int64),
("MediaType", ctypes.c_ulong),
("TracksPerCylinder", ctypes.c_ulong),
("SectorsPerTrack", ctypes.c_ulong),
("BytesPerSector", ctypes.c_ulong)]
# 打开磁盘驱动器
handle = ctypes.windll.kernel32.CreateFileW(
ctypes.c_wchar_p("\\\\.\\PhysicalDrive0"),
GENERIC_READ | GENERIC_WRITE,
FILE_SHARE_READ | FILE_SHARE_WRITE,
None,
OPEN_EXISTING,
0,
None)
# 获取磁盘几何信息
disk_geometry = DISK_GEOMETRY()
bytes_returned = ctypes.c_ulong()
ctypes.windll.kernel32.DeviceIoControl(
handle,
IOCTL_DISK_GET_DRIVE_GEOMETRY,
None,
0,
ctypes.byref(disk_geometry),
ctypes.sizeof(disk_geometry),
ctypes.byref(bytes_returned),
None)
# 打印磁盘几何信息
print("Cylinders: ", disk_geometry.Cylinders)
print("MediaType: ", disk_geometry.MediaType)
print("TracksPerCylinder: ", disk_geometry.TracksPerCylinder)
print("SectorsPerTrack: ", disk_geometry.SectorsPerTrack)
print("BytesPerSector: ", disk_geometry.BytesPerSector)
# 关闭磁盘驱动器
ctypes.windll.kernel32.CloseHandle(handle)
```
这段代码打开了PhysicalDrive0这个磁盘驱动器,并获取了磁盘几何信息。可以根据需要修改代码中的常量和结构体。
阅读全文
相关推荐
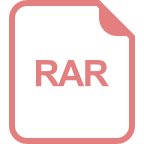
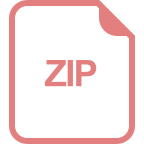
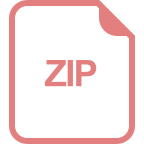
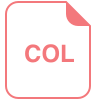


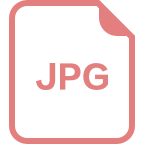