C#设计一个复制文件的进度条
时间: 2023-06-19 22:08:30 浏览: 64
以下是使用C#编写的复制文件的进度条示例代码:
```csharp
using System;
using System.IO;
using System.Windows.Forms;
public class FileCopyProgressBar : Form
{
private ProgressBar progressBar;
private Button startButton;
public FileCopyProgressBar()
{
Text = "File Copy Progress Bar";
progressBar = new ProgressBar();
progressBar.Dock = DockStyle.Top;
Controls.Add(progressBar);
startButton = new Button();
startButton.Text = "Copy File";
startButton.Dock = DockStyle.Bottom;
startButton.Click += new EventHandler(OnStartButtonClicked);
Controls.Add(startButton);
}
private void OnStartButtonClicked(object sender, EventArgs e)
{
string sourceFilePath = "path_to_source_file";
string destinationFilePath = "path_to_destination_file";
// Open the source file for reading
using (FileStream sourceStream = new FileStream(sourceFilePath, FileMode.Open))
{
// Create the destination file
using (FileStream destinationStream = new FileStream(destinationFilePath, FileMode.Create))
{
// Set the buffer size to 10KB
byte[] buffer = new byte[10240];
int bytesRead;
long totalBytesRead = 0;
long fileSize = sourceStream.Length;
// Copy the file and update the progress bar
while ((bytesRead = sourceStream.Read(buffer, 0, buffer.Length)) > 0)
{
destinationStream.Write(buffer, 0, bytesRead);
totalBytesRead += bytesRead;
int percentComplete = (int)((double)totalBytesRead / fileSize * 100);
progressBar.Value = percentComplete;
Application.DoEvents();
}
}
}
MessageBox.Show("File copied successfully!");
}
public static void Main()
{
Application.Run(new FileCopyProgressBar());
}
}
```
在上面的示例代码中,我们创建了一个名为`FileCopyProgressBar`的窗体,其中包含一个`ProgressBar`和一个`Button`。当用户单击`Button`时,我们将复制源文件到目标文件,并在进程中更新进度条。请注意,我们使用`Application.DoEvents()`来允许UI更新。
相关推荐
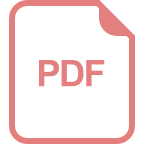
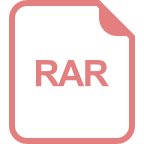
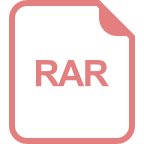














