要求开发手机系统软件中的一个子功能,实现从手机短信内容中识别和提取电话号码(7位或8位)和手机号码(11位),并将其存入通讯录中。2.设计分析要从手机短信的内容中识别电话号码或手机号码,必须从短信的第一个字符开始查找,找到第一个数值型字符(‘0’~‘9’),然后依次判断其后的字符,若其后有连续的6个或7个数值型字符,则将其识别成电话号码并提取,若其后有连续的11个数值型字符,则将其识别成手机号码并提取。继续向后搜索直到整个短信查找完毕。3.存储结构类型定义①短信的存储结构类型定义 typedef struct{char word[200];/*短信内容*/int length; /*短信长度*/}Message;②通讯录中记录的存储结构类型的定义typedef struct{char name[8]; /*姓名*/char phone[11]; /*电话号码或手机号码*/}Note;
时间: 2024-02-28 08:53:48 浏览: 107
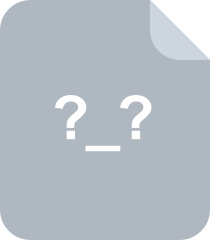
手机短信内容中电话号码和手机号码的识别与提取

好的,您的要求是实现从手机短信中识别和提取电话号码和手机号码,并将其存入通讯录中。根据您提供的设计分析,我可以给您提供以下思路:
1. 首先,您需要读取手机短信,并将其存储到一个 Message 结构体中,结构体中包含短信内容和短信长度。
2. 然后,您需要从短信的第一个字符开始查找,找到第一个数值型字符(‘0’~‘9’),然后依次判断其后的字符,若其后有连续的6个或7个数值型字符,则将其识别成电话号码并提取,若其后有连续的11个数值型字符,则将其识别成手机号码并提取。
3. 将识别出的电话号码或手机号码存储到 Note 结构体中,结构体中包含姓名和电话号码或手机号码。
4. 最后,将 Note 结构体中的信息存储到通讯录中。
以下是该功能的 C 语言代码示例:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX_SMS_LENGTH 200
#define MAX_NAME_LENGTH 8
#define MAX_PHONE_LENGTH 11
typedef struct {
char word[MAX_SMS_LENGTH]; /*短信内容*/
int length; /*短信长度*/
} Message;
typedef struct {
char name[MAX_NAME_LENGTH]; /*姓名*/
char phone[MAX_PHONE_LENGTH]; /*电话号码或手机号码*/
} Note;
void extract_phone_number(Message* message, Note* note) {
int start = 0;
int phone_start = -1;
int phone_end = -1;
while (start < message->length) {
if (message->word[start] >= '0' && message->word[start] <= '9') {
phone_start = start;
phone_end = start;
start++;
while (start < message->length && message->word[start] >= '0' && message->word[start] <= '9') {
phone_end = start;
start++;
}
if (phone_end - phone_start == 6 || phone_end - phone_start == 7) {
strncpy(note->phone, message->word + phone_start, phone_end - phone_start + 1);
note->phone[phone_end - phone_start + 1] = '\0';
} else if (phone_end - phone_start == 10 || phone_end - phone_start == 11) {
strncpy(note->phone, message->word + phone_start, phone_end - phone_start + 1);
note->phone[phone_end - phone_start + 1] = '\0';
}
if (strlen(note->phone) > 0) {
break;
}
} else {
start++;
}
}
}
void save_to_contact(Note* note) {
// 将 note 存储到通讯录中
}
int main() {
Message message;
Note note;
// 读取短信
fgets(message.word, MAX_SMS_LENGTH, stdin);
message.length = strlen(message.word) - 1;
message.word[message.length] = '\0';
memset(note.name, 0, MAX_NAME_LENGTH);
memset(note.phone, 0, MAX_PHONE_LENGTH);
extract_phone_number(&message, ¬e);
save_to_contact(¬e);
return 0;
}
```
以上是一个简单的示例,您可以根据您的实际情况进行修改和完善。
阅读全文
相关推荐
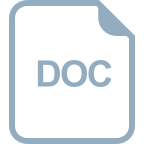
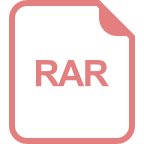
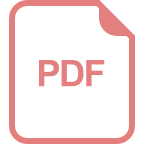
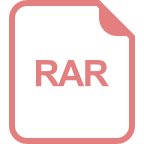
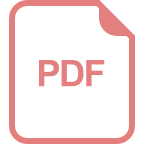
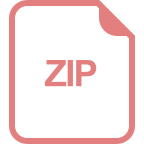
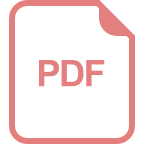
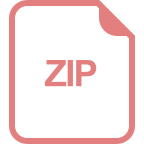
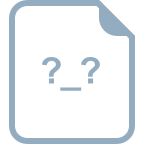
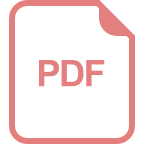
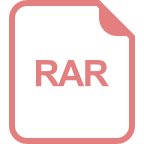
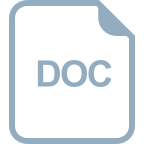
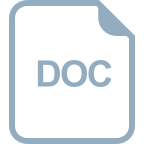
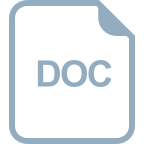
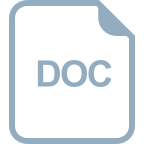
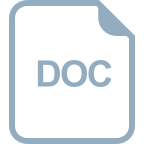
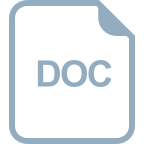