android开发 OpenVPN 实例代码
时间: 2023-09-23 10:10:16 浏览: 85
以下是一个简单的 Android 应用程序,它使用 OpenVPN 与远程服务器进行连接。该应用程序包含了一个 OpenVPN 配置文件和一些基本的 UI 元素,以便用户可以输入其凭据等信息。
首先,需要添加以下依赖项到 app 的 build.gradle 文件:
```
dependencies {
implementation 'com.github.schwabe:ics-openvpn:0.7.22'
}
```
接下来,需要创建一个 OpenVPN 配置文件。可以使用 OpenVPN 客户端生成一个配置文件,或手动创建一个。以下是一个示例配置文件:
```
dev tun
client
proto udp
remote yourserver.com 1194
resolv-retry infinite
nobind
persist-key
persist-tun
ca ca.crt
cert client.crt
key client.key
tls-auth ta.key 1
cipher AES-256-CBC
auth SHA256
comp-lzo
verb 3
```
将上面的配置文件保存在 app/src/main/res/raw 目录下,例如命名为 `config.ovpn`。
接下来,需要创建一个 UI,以便用户可以输入其凭据并启动连接。以下是一个示例布局:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<EditText
android:id="@+id/username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Username"/>
<EditText
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Password"
android:inputType="textPassword"/>
<Button
android:id="@+id/connect_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Connect"/>
</RelativeLayout>
```
在 Activity 中,需要将 OpenVPN 配置文件读入内存,并准备传递给 OpenVPN API。还需要处理连接按钮的点击事件,并使用提供的凭据设置 OpenVPN API 的身份验证信息。以下是一个示例 Activity:
```
import android.content.res.Resources;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import java.io.InputStream;
import de.blinkt.openvpn.OpenVpnConfig;
import de.blinkt.openvpn.core.VpnStatus;
import de.blinkt.openvpn.core.VpnStatus.StateListener;
import de.blinkt.openvpn.core.VpnThread;
public class MainActivity extends AppCompatActivity implements StateListener {
private static final String TAG = "MainActivity";
private EditText mUsernameEditText;
private EditText mPasswordEditText;
private Button mConnectButton;
private OpenVpnConfig mVpnConfig;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mUsernameEditText = findViewById(R.id.username);
mPasswordEditText = findViewById(R.id.password);
mConnectButton = findViewById(R.id.connect_button);
Resources resources = getResources();
InputStream inputStream = resources.openRawResource(R.raw.config);
byte[] buffer = new byte[inputStream.available()];
inputStream.read(buffer);
mVpnConfig = new OpenVpnConfig(buffer);
mConnectButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String username = mUsernameEditText.getText().toString();
String password = mPasswordEditText.getText().toString();
if (!username.isEmpty() && !password.isEmpty()) {
mVpnConfig.setAuthUserPass(username, password);
}
startVpn();
}
});
}
private void startVpn() {
VpnThread.start(mVpnConfig, this);
}
@Override
public void onUpdateState(String state, String logmessage, int localizedResId, StateListener.Reason reason) {
// Handle state updates here
}
}
```
在 onCreate() 方法中,读取配置文件并设置按钮的点击事件处理程序。在点击事件处理程序中,获取用户输入的凭据并将其设置为 OpenVPN API 的身份验证信息。最后,调用 startVpn() 方法以启动连接。
在 onUpdateState() 方法中,可以处理 OpenVPN 状态更新。例如,可以更新 UI 来显示连接状态,并在连接断开时通知用户。
相关推荐
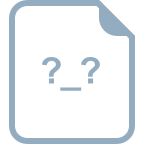
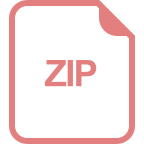














