python中的Crypto加密
时间: 2024-04-07 08:33:46 浏览: 18
Python中的加密模块是`cryptography`,它提供了丰富的加密算法和功能。你可以使用这个模块进行对称加密、非对称加密、哈希函数的计算等。
对称加密是指使用同一个密钥进行加密和解密的方式,常见的对称加密算法有AES和DES等。你可以使用`cryptography`模块中的`Fernet`类来进行对称加密操作。以下是一个使用AES算法进行加密和解密的示例:
```python
from cryptography.fernet import Fernet
# 生成密钥
key = Fernet.generate_key()
# 创建Fernet对象
cipher = Fernet(key)
# 加密数据
data = b"Hello, World!"
encrypted_data = cipher.encrypt(data)
# 解密数据
decrypted_data = cipher.decrypt(encrypted_data)
print(decrypted_data.decode()) # 输出: Hello, World!
```
非对称加密是指使用一对密钥进行加密和解密的方式,常见的非对称加密算法有RSA和ECC等。你可以使用`cryptography`模块中的`RSA`类来进行非对称加密操作。以下是一个使用RSA算法进行密钥生成、加密和解密的示例:
```python
from cryptography.hazmat.primitives import serialization
from cryptography.hazmat.primitives.asymmetric import rsa
# 生成RSA密钥对
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048
)
public_key = private_key.public_key()
# 将私钥和公钥保存为PEM格式的文件
with open('private_key.pem', 'wb') as f:
f.write(
private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.PKCS8,
encryption_algorithm=serialization.NoEncryption()
)
)
with open('public_key.pem', 'wb') as f:
f.write(
public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
)
# 加载私钥和公钥
private_key = serialization.load_pem_private_key(
open('private_key.pem', 'rb').read(),
password=None
)
public_key = serialization.load_pem_public_key(
open('public_key.pem', 'rb').read()
)
# 加密数据
data = b"Hello, World!"
encrypted_data = public_key.encrypt(
data,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
# 解密数据
decrypted_data = private_key.decrypt(
encrypted_data,
padding.OAEP(
mgf=padding.MGF1(algorithm=hashes.SHA256()),
algorithm=hashes.SHA256(),
label=None
)
)
print(decrypted_data.decode()) # 输出: Hello, World!
```
除了对称加密和非对称加密之外,`cryptography`模块还提供了丰富的哈希函数计算功能,用于生成数据的唯一摘要。你可以使用`cryptography`模块中的`hashes`类来进行哈希函数计算。以下是一个使用SHA256算法计算哈希值的示例:
```python
from cryptography.hazmat.primitives import hashes
# 计算哈希值
data = b"Hello, World!"
digest = hashes.Hash(hashes.SHA256())
digest.update(data)
hash_value = digest.finalize()
print(hash_value.hex()) # 输出: 7d711d5c0b5c8f9e20d15c6489a2b6036c5f0683e6e63e8b5a7bf8ef646a7e45
```
这只是`cryptography`模块的一部分功能,你可以根据自己的需求进一步探索和使用其中的加密算法和功能。
相关推荐
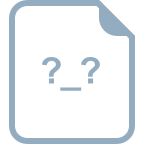
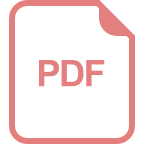
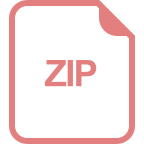














