window c++ 如何读取已存在的共享内存中的数据
时间: 2024-05-15 15:13:45 浏览: 90
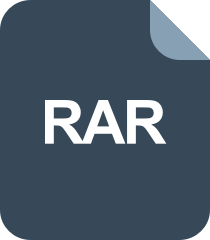
C++操作共享内存读写代码

在Windows C++中,读取已存在的共享内存中的数据需要经过以下步骤:
1. 使用 `OpenFileMapping` 函数打开共享内存对象,该函数返回一个句柄。
2. 使用 `MapViewOfFile` 函数将共享内存映射到当前进程的地址空间中,该函数返回指向共享内存的指针。
3. 通过指针访问共享内存中的数据。
4. 使用 `UnmapViewOfFile` 函数取消映射。
5. 使用 `CloseHandle` 函数关闭句柄。
下面是一个示例代码,展示如何读取已存在的共享内存中的数据:
```c++
#include <iostream>
#include <Windows.h>
int main()
{
// 打开共享内存对象
HANDLE hMapFile = OpenFileMapping(FILE_MAP_READ, FALSE, L"mySharedMemory");
if (hMapFile == NULL)
{
std::cout << "OpenFileMapping failed" << std::endl;
return 1;
}
// 将共享内存映射到当前进程的地址空间中
LPVOID lpMapAddress = MapViewOfFile(hMapFile, FILE_MAP_READ, 0, 0, 0);
if (lpMapAddress == NULL)
{
std::cout << "MapViewOfFile failed" << std::endl;
CloseHandle(hMapFile);
return 1;
}
// 读取共享内存中的数据
int* pData = (int*)lpMapAddress;
std::cout << "Data read from shared memory: " << *pData << std::endl;
// 取消映射
UnmapViewOfFile(lpMapAddress);
// 关闭句柄
CloseHandle(hMapFile);
return 0;
}
```
在上面的代码中,假设共享内存对象的名称为 `"mySharedMemory"`,且共享内存中存储的是一个整数。程序通过 `OpenFileMapping` 函数打开共享内存对象,并使用 `MapViewOfFile` 函数将共享内存映射到当前进程的地址空间中。随后,程序通过指针访问共享内存中的数据,最后取消映射并关闭句柄。
阅读全文
相关推荐


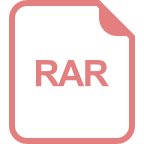
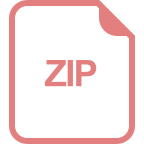
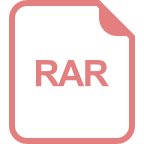
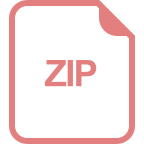
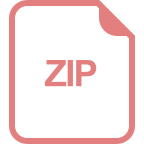
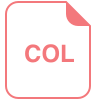
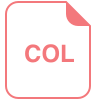
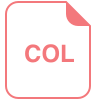
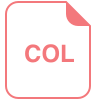
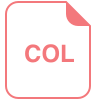
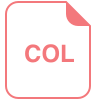
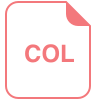