public class MobileShop { InnerPurchaseMoney purchaseMoney1 = new InnerPurchaseMoney(20000); InnerPurchaseMoney purchaseMoney2 = new InnerPurchaseMoney(10000); int mobileAmount = 0; public MobileShop() { } public void setmobileAmount(int mobileAmount) { this.mobileAmount = mobileAmount; } public int getmobileAmount() { return this.mobileAmount; } class InnerPurchaseMoney{ int moneyValue = 0; public InnerPurchaseMoney(int moneyValue) { this.moneyValue = moneyValue; } public void buyMobile() { if(moneyValue>=20000) { mobileAmount -= 6; System.out.println("并输出使用价值为20000的内部购物券购买了6部手机。"); } else if(moneyValue>=10000 && moneyValue<20000) { mobileAmount -= 3; System.out.println("并输出使用价值为10000的内部购物券购买了3部手机。"); } } } }在buyMobile方法中判断,如果优惠券的价值为负数,则抛出非法参数异常,并采用两种方法(抛出和处理)对该异常进行处理。
时间: 2024-03-11 07:46:51 浏览: 23
可以在 `buyMobile()` 方法中添加以下代码来判断优惠券的价值是否为负数,并抛出非法参数异常:
```
if(moneyValue < 0) {
throw new IllegalArgumentException("优惠券的价值不能为负数!");
}
```
这样,如果优惠券的价值为负数,就会抛出非法参数异常。
处理该异常可以采用两种方法:
1. 在 `buyMobile()` 方法中使用 try-catch 块捕获该异常,然后在 catch 块中打印异常信息或进行其他处理。例如:
```
public void buyMobile() {
try {
if(moneyValue < 0) {
throw new IllegalArgumentException("优惠券的价值不能为负数!");
}
if(moneyValue>=20000) {
mobileAmount -= 6;
System.out.println("并输出使用价值为20000的内部购物券购买了6部手机。");
}
else if(moneyValue>=10000 && moneyValue<20000) {
mobileAmount -= 3;
System.out.println("并输出使用价值为10000的内部购物券购买了3部手机。");
}
} catch (IllegalArgumentException e) {
System.out.println("非法参数异常:" + e.getMessage());
// 或者进行其他处理
}
}
```
2. 在调用 `buyMobile()` 方法的地方使用 try-catch 块捕获该异常,然后在 catch 块中打印异常信息或进行其他处理。例如:
```
MobileShop shop = new MobileShop();
shop.setmobileAmount(10);
MobileShop.InnerPurchaseMoney coupon = shop.new InnerPurchaseMoney(-500);
try {
coupon.buyMobile();
} catch (IllegalArgumentException e) {
System.out.println("非法参数异常:" + e.getMessage());
// 或者进行其他处理
}
```
相关推荐




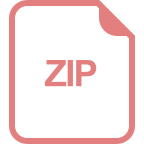
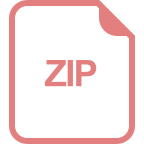
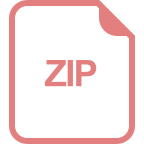
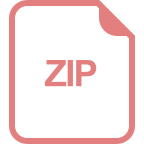
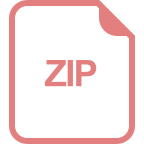
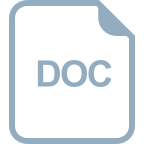
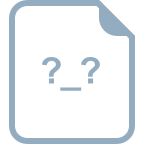
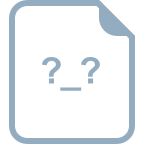