使用vue2封装一个蓝色按钮组件
时间: 2023-08-31 11:35:58 浏览: 111
### 回答1:
可以使用Vue官方提供的API来封装一个蓝色按钮组件,具体来说,可以创建一个名为BlueButton.vue的文件,在文件中使用template标签来定义HTML结构,style标签定义按钮的样式,并使用script标签定义按钮的功能,最后导出按钮组件。
### 回答2:
Vue2是目前非常流行的前端框架之一,它提供了一种方便的方式来封装可复用的组件。下面是一个封装蓝色按钮组件的示例代码:
```html
<template>
<button class="blue-button">
<slot></slot>
</button>
</template>
<script>
export default {
name: 'BlueButton',
// 组件的props定义
props: {
// 可以接受一个自定义的class
class: {
type: String,
default: ''
}
},
// 组件的样式
style: `
.blue-button {
width: 100px;
height: 40px;
background-color: blue;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
`
}
</script>
<style scoped>
/* 使用scoped属性将样式限制在当前组件中 */
.blue-button {
width: 100px;
height: 40px;
background-color: blue;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
</style>
```
使用示例:
```html
<template>
<div>
<blue-button>Click Me</blue-button>
</div>
</template>
<script>
import BlueButton from './BlueButton.vue'
export default {
components: {
BlueButton
}
}
</script>
```
这样就可以在Vue项目中使用`<blue-button></blue-button>`来渲染一个蓝色的按钮,并且按钮的文本内容可以自定义。按钮的样式也可以进一步自定义,通过传入一个自定义的class来覆盖默认样式。
### 回答3:
Vue是一个流行的JavaScript框架,用于构建用户界面。通过Vue的组件化系统,我们可以封装可重用的蓝色按钮组件。
首先,我们需要创建一个Vue组件。我们可以使用Vue的单文件组件(.vue)来封装我们的按钮组件。
在.vue文件中,我们可以定义模板、样式和逻辑。以下是一个示例蓝色按钮组件的代码:
```html
<template>
<button class="blue-button" @click="handleClick">
{{ buttonText }}
</button>
</template>
<script>
export default {
props: {
buttonText: {
type: String,
default: 'Click me' // 默认按钮文本
}
},
methods: {
handleClick() {
// 处理按钮点击事件
console.log('Button clicked');
}
}
}
</script>
<style scoped>
.blue-button {
background-color: blue;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
</style>
```
在上述示例中,我们定义了一个名为`blue-button`的按钮组件。该组件接受一个`buttonText`属性,用于设置按钮的文本。当按钮被点击时,将触发`handleClick`方法,控制台将输出“Button clicked”。我们还为按钮应用了一些样式,使其具有蓝色的背景和白色的文本。
要在应用中使用我们的蓝色按钮组件,需要在父组件中导入它,并在模板中使用它。以下是一个使用示例:
```html
<template>
<div>
<blue-button buttonText="Click me"></blue-button>
</div>
</template>
<script>
import BlueButton from './BlueButton.vue';
export default {
components: {
BlueButton
}
}
</script>
```
在上述示例中,我们将蓝色按钮组件引入为`BlueButton`,然后在模板中使用它,将`buttonText`属性设置为“Click me”。
这样,我们就成功封装了一个蓝色按钮组件,并在应用中使用它。无论我们需要在应用的哪个地方使用蓝色按钮,只需要简单地将`<blue-button>`标签添加到模板中即可。
相关推荐
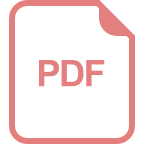
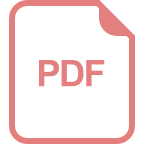














