SpringBoot SSE自定义事件
时间: 2024-12-28 07:23:45 浏览: 7
### 实现Spring Boot中的SSE自定义事件
在Spring Boot中实现服务器发送事件(Server-Sent Events, SSE),可以创建一个控制器来处理HTTP请求并返回`TextEventStream`对象。这允许浏览器或其他客户端订阅来自服务端的实时更新。
#### 创建SSE Controller
为了支持SSE,需编写如下所示的一个简单的REST控制器:
```java
import org.springframework.http.MediaType;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Flux;
@RestController
@RequestMapping("/events")
public class EventController {
@GetMapping(value = "/custom", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<String> streamCustomEvents() {
return Flux.interval(Duration.ofSeconds(1))
.map(sequence -> "event-" + sequence);
}
}
```
此代码片段展示了如何通过Reactor库生成每秒一次的时间间隔,并将其映射成字符串形式的消息流[^1]。
对于更复杂的场景,比如当希望推送特定类型的Java对象作为事件数据时,则可采用泛型化的方式封装这些消息体:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
// ... other imports ...
@RestController
@RequestMapping("/events")
public class CustomEventController {
private final ObjectMapper objectMapper = new ObjectMapper();
record CustomEvent(String type, String message) {}
@GetMapping(value = "/complex", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<ServerSentEvent<?>> sendComplexEvents() {
var events = List.of(
new CustomEvent("info", "This is an informational event"),
new CustomEvent("warning", "Be cautious!")
);
return Flux.fromIterable(events).delayElements(Duration.ofSeconds(2))
.map(event -> ServerSentEvent.<String>builder()
.id(UUID.randomUUID().toString())
.data(() -> objectMapper.writeValueAsString(event))
.build());
}
}
```
这段代码实现了更加结构化的事件传输机制,其中包含了不同种类的信息以及警告级别的通知。这里使用了Jackson库来进行JSON序列化操作。
请注意,在实际应用开发过程中还需要考虑连接管理、错误恢复策略等问题以确保系统的健壮性和稳定性。
阅读全文
相关推荐
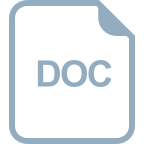
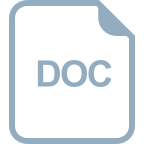
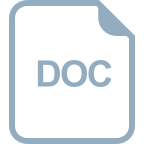
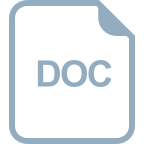
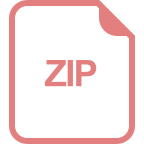
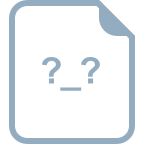
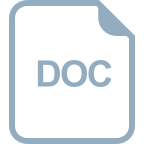


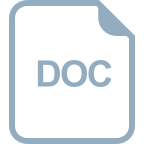
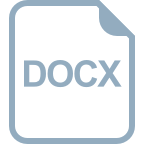
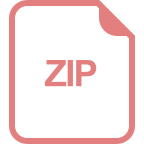
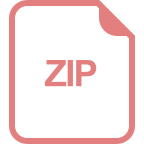
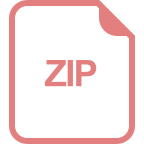
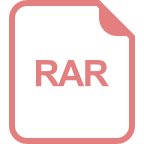
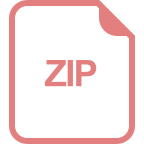
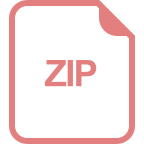