from fnmatch import fnmatch basedir = r"D:\MY python\English" for root, dirs, files in os.walk(basedir): for file in files: if fnmatch(file, "*.mp3"): english3 = os.path.join(root, file) print(english3)
时间: 2024-04-08 07:31:55 浏览: 169
这段代码是用来遍历指定目录下的文件,并打印出所有以 `.mp3` 结尾的文件路径。它使用了 `os.walk` 函数来递归遍历目录树,获取每个目录下的文件和子目录。对于每个文件,它使用了 `fnmatch` 函数来判断文件名是否匹配指定的模式(这里是 `"*.mp3"`),如果匹配则打印出文件的路径。
代码的逻辑看起来是正确的,并且会输出所有以 `.mp3` 结尾的文件路径。如果你想遍历其他类型的文件,只需要修改 `fnmatch` 函数中的模式即可。
如果你有任何其他问题,请随时提问!
相关问题
from fnmatch import fnmatch basedir = r"D:\MY python\English" for root, dirs, files in os.walk(basedir): for file in files: english3 = os.path.join(root, file) if fnmatch(file, f"{english1}*.mp3"): print(english3)
根据你提供的代码,我注意到你导入了`fnmatch`模块,并使用了`fnmatch`函数来进行文件名的模式匹配。另外,你定义了一个名为`basedir`的变量来指定要遍历的目录。
然而,我还是注意到了一个问题:`english1`变量在代码中没有定义。如果你想使用`english1`作为匹配模式的一部分,请确保在使用之前已经定义了该变量。
如果你想遍历目录下所有以`.mp3`结尾的文件,可以将代码修改为:
```python
import os
from fnmatch import fnmatch
basedir = r"D:\MY python\English"
for root, dirs, files in os.walk(basedir):
for file in files:
if fnmatch(file, "*.mp3"):
english3 = os.path.join(root, file)
print(english3)
```
这样就会输出所有以`.mp3`结尾的文件路径。
希望这次能够满足你的需求。如果还有其他问题,请随时提问!
import os import random import time from fnmatch import fnmatch import pygame import tkinter as tk root = tk.Tk() root.geometry("450x200+374+182") root.title("英语单词") english1 = "" def word(): while 1: path = "D:\MY python\English" lists = os.listdir(path) english = (random.choice(lists)) global english1 english1 = english.strip(".mp3") print(english1) time.sleep(3) basedir = r"D:\MY python\English" for root, dirs, files in os.walk(basedir): for file in files: english3 = os.path.join(root, file) if fnmatch(file, f"{english1}*.mp3"): pygame.mixer.init() pygame.mixer.music.load(english3) pygame.mixer.music.play() time.sleep(3) button = tk.Button(root, text="开始", command=word) button.grid(row=0, column=0) w = tk.Label(root, text=f"{english1}") w.grid(row=1, column=0) root.mainloop()
这段代码是一个简单的英语单词学习程序。它使用了`os`、`random`、`time`、`fnmatch`、`pygame`和`tkinter`库。通过点击"开始"按钮,程序会从指定路径中随机选择一个英语单词的音频文件,并播放出来。同时,程序会将选择的单词显示在窗口上方的标签中。
需要注意的是,这段代码中使用了硬编码的路径,如果你想要运行该程序,需要根据你自己的文件路径进行相应的修改。
阅读全文
相关推荐
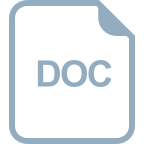
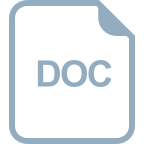
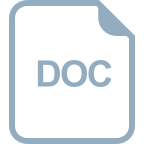

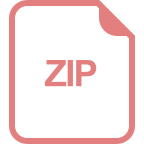
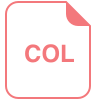
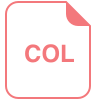
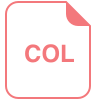
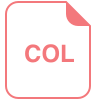







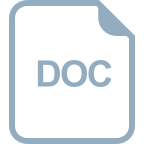